|
2 | 2 |
|
3 | 3 | [Etherscan](https://etherscan.io/apis) Java API implementation.
|
4 | 4 |
|
| 5 | +Library supports all available EtherScan *API* calls for all available *Ethereum Networks*. |
5 | 6 |
|
6 | 7 | 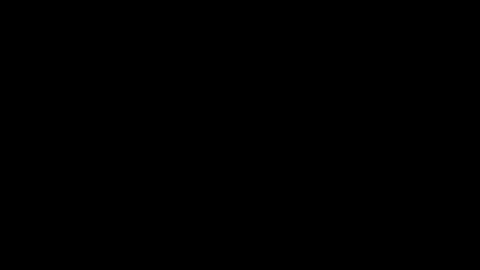
|
7 | 8 |
|
@@ -34,55 +35,107 @@ dependencies {
|
34 | 35 | - [Transactions](#transaction-api)
|
35 | 36 | - [Version History](#version-history)
|
36 | 37 |
|
37 |
| -## Overall |
38 |
| - |
39 |
| -How all is linked together: |
40 |
| - |
41 | 38 | ## Api Examples
|
42 | 39 |
|
43 | 40 | You can read about all API methods on [Etherscan](https://etherscan.io/apis)
|
44 | 41 |
|
45 |
| -You can you API with you key or without key as well. |
| 42 | +*Library support all available EtherScan API.* |
| 43 | + |
| 44 | +You can use API with you key or without key as well (Check API request\sec restrictions). |
46 | 45 | ```java
|
47 | 46 | EtherScanApi api = new EtherScanApi();
|
48 | 47 | EtherScanApi api = new EtherScanApi("YourApiKey");
|
49 | 48 | ```
|
50 | 49 |
|
| 50 | +Below there are examples for each API category. |
| 51 | + |
51 | 52 | ### Mainnet and Testnets
|
52 |
| -API support: *MAINNET, ROPSTEN, KOVAN, RINKEBY* networks. |
| 53 | +API support Ethereum: *[MAINNET](https://etherscan.io), [ROPSTEN](https://ropsten.etherscan.io), [KOVAN](https://kovan.etherscan.io), [RINKEBY](https://rinkeby.etherscan.io)* networks. |
53 | 54 | ```java
|
54 | 55 | EtherScanApi api = new EtherScanApi(EthNetwork.MAINNET);
|
55 | 56 | EtherScanApi api = new EtherScanApi("YourApiKey", EthNetwork.KOVAN);
|
56 | 57 | ```
|
57 | 58 |
|
58 | 59 | ### Account Api
|
59 |
| -**Get Ether Balance for a single Address Example** |
| 60 | +**Get Ether Balance for a single Address** |
60 | 61 | ```java
|
61 | 62 | EtherScanApi api = new EtherScanApi();
|
62 | 63 | Balance balance = api.account().balance("0x8d4426f94e42f721C7116E81d6688cd935cB3b4F");
|
63 | 64 | ```
|
64 | 65 |
|
65 | 66 | ### Block Api
|
| 67 | +**Get uncles block for block height** |
| 68 | +```java |
| 69 | +EtherScanApi api = new EtherScanApi(); |
| 70 | +Optional<UncleBlock> uncles = api.block().uncles(200000); |
| 71 | +``` |
66 | 72 |
|
67 | 73 | ### Contract Api
|
| 74 | +**Request contract ABI from [verified codes](https://etherscan.io/contractsVerified)** |
| 75 | +```java |
| 76 | +EtherScanApi api = new EtherScanApi(); |
| 77 | +Abi abi = api.contract().contractAbi("0xBB9bc244D798123fDe783fCc1C72d3Bb8C189413"); |
| 78 | +``` |
68 | 79 |
|
69 | 80 | ### Logs Api
|
| 81 | +**Get event logs for single topic** |
| 82 | +```java |
| 83 | +EtherScanApi api = new EtherScanApi(); |
| 84 | +LogQuery query = LogQueryBuilder.with("0x33990122638b9132ca29c723bdf037f1a891a70c") |
| 85 | + .topic("0xf63780e752c6a54a94fc52715dbc5518a3b4c3c2833d301a204226548a2a8545") |
| 86 | + .build(); |
| 87 | +List<Log> logs = api.logs().logs(query); |
| 88 | +``` |
| 89 | + |
| 90 | +**Get event logs for 3 topics with respectful operations** |
| 91 | +```java |
| 92 | +EtherScanApi api = new EtherScanApi(); |
| 93 | +LogQuery query = LogQueryBuilder.with("0x33990122638b9132ca29c723bdf037f1a891a70c", 379224, 400000) |
| 94 | + .topic("0xf63780e752c6a54a94fc52715dbc5518a3b4c3c2833d301a204226548a2a8545", |
| 95 | + "0x72657075746174696f6e00000000000000000000000000000000000000000000", |
| 96 | + "0x72657075746174696f6e00000000000000000000000000000000000000000000") |
| 97 | + .setOpTopic0_1(LogOp.AND) |
| 98 | + .setOpTopic0_2(LogOp.OR) |
| 99 | + .setOpTopic1_2(LogOp.AND) |
| 100 | + .build(); |
| 101 | +List<Log> logs = api.logs().logs(query); |
| 102 | +``` |
70 | 103 |
|
71 | 104 | ### Proxy Api
|
| 105 | +**Get tx detailds with proxy endpoint** |
| 106 | +```java |
| 107 | +EtherScanApi api = new EtherScanApi(EthNetwork.MAINNET); |
| 108 | +Optional<TxProxy> tx = api.proxy().tx("0x1e2910a262b1008d0616a0beb24c1a491d78771baa54a33e66065e03b1f46bc1"); |
| 109 | +``` |
| 110 | + |
| 111 | +**Get block info with proxy endpoint** |
| 112 | +```java |
| 113 | +EtherScanApi api = new EtherScanApi(EthNetwork.MAINNET); |
| 114 | +Optional<BlockProxy> block = api.proxy().block(15215); |
| 115 | +``` |
72 | 116 |
|
73 | 117 | ### Stats Api
|
| 118 | +**Statistic about last price** |
| 119 | +```java |
| 120 | +EtherScanApi api = new EtherScanApi(); |
| 121 | +Price price = api.stats().lastPrice(); |
| 122 | +``` |
74 | 123 |
|
75 | 124 | ### Transaction Api
|
76 |
| - |
| 125 | +**Request receipt status for tx** |
| 126 | +```java |
| 127 | +EtherScanApi api = new EtherScanApi(); |
| 128 | +Optional<Boolean> status = api.txs().receiptStatus("0x513c1ba0bebf66436b5fed86ab668452b7805593c05073eb2d51d3a52f480a76"); |
| 129 | +``` |
77 | 130 |
|
78 | 131 | ### Token Api
|
79 | 132 | You can read account API [here](https://etherscan.io/apis#accounts)
|
80 | 133 |
|
81 |
| -Token API migrated to account & stats respectfully. |
| 134 | +Token API methods migrated to [Account](#account-api) & [Stats](#stats-api) respectfully. |
82 | 135 |
|
83 | 136 | ## Version History
|
84 | 137 |
|
85 |
| -**1.0.0** - Initial project with all API functionality. |
| 138 | +**1.0.0** - Initial project with all API functionality, for all available networks, with tests coverage for all cases. |
86 | 139 |
|
87 | 140 | ## License
|
88 | 141 |
|
|
0 commit comments