diff --git a/packages/core/src/blocks/ListItemBlockContent/NumberedListItemBlockContent/NumberedListItemBlockContent.ts b/packages/core/src/blocks/ListItemBlockContent/NumberedListItemBlockContent/NumberedListItemBlockContent.ts
index 6c0f72a0a0..37e04beb27 100644
--- a/packages/core/src/blocks/ListItemBlockContent/NumberedListItemBlockContent/NumberedListItemBlockContent.ts
+++ b/packages/core/src/blocks/ListItemBlockContent/NumberedListItemBlockContent/NumberedListItemBlockContent.ts
@@ -126,7 +126,7 @@ const NumberedListItemBlockContent = createStronglyTypedTiptapNode({
const startIndex =
parseInt(parent.getAttribute("start") || "1") || 1;
- if (element.previousSibling || startIndex === 1) {
+ if (element.previousElementSibling || startIndex === 1) {
return {};
}
diff --git a/packages/core/src/blocks/defaultProps.ts b/packages/core/src/blocks/defaultProps.ts
index 4fb0b838c8..3a596b880b 100644
--- a/packages/core/src/blocks/defaultProps.ts
+++ b/packages/core/src/blocks/defaultProps.ts
@@ -1,3 +1,5 @@
+import { Attribute } from "@tiptap/core";
+
import type { Props, PropSchema } from "../schema/index.js";
// TODO: this system should probably be moved / refactored.
@@ -22,3 +24,54 @@ export type DefaultProps = Props;
// `blockContent` nodes. Ensures that they are not redundantly added to
// a custom block's TipTap node attributes.
export const inheritedProps = ["backgroundColor", "textColor"];
+
+export const getBackgroundColorAttribute = (
+ attributeName = "backgroundColor"
+): Attribute => ({
+ default: defaultProps.backgroundColor.default,
+ parseHTML: (element) => {
+ if (element.hasAttribute("data-background-color")) {
+ return element.getAttribute("data-background-color");
+ }
+
+ if (element.style.backgroundColor) {
+ return element.style.backgroundColor;
+ }
+
+ return defaultProps.backgroundColor.default;
+ },
+ renderHTML: (attributes) => {
+ if (attributes[attributeName] === defaultProps.backgroundColor.default) {
+ return {};
+ }
+
+ return {
+ "data-background-color": attributes[attributeName],
+ };
+ },
+});
+
+export const getTextColorAttribute = (
+ attributeName = "textColor"
+): Attribute => ({
+ default: defaultProps.textColor.default,
+ parseHTML: (element) => {
+ if (element.hasAttribute("data-text-color")) {
+ return element.getAttribute("data-text-color");
+ }
+
+ if (element.style.color) {
+ return element.style.color;
+ }
+
+ return defaultProps.textColor.default;
+ },
+ renderHTML: (attributes) => {
+ if (attributes[attributeName] === defaultProps.textColor.default) {
+ return {};
+ }
+ return {
+ "data-text-color": attributes[attributeName],
+ };
+ },
+});
diff --git a/packages/core/src/extensions/BackgroundColor/BackgroundColorExtension.ts b/packages/core/src/extensions/BackgroundColor/BackgroundColorExtension.ts
index fca4922a1a..61a8b8c14b 100644
--- a/packages/core/src/extensions/BackgroundColor/BackgroundColorExtension.ts
+++ b/packages/core/src/extensions/BackgroundColor/BackgroundColorExtension.ts
@@ -1,5 +1,5 @@
import { Extension } from "@tiptap/core";
-import { defaultProps } from "../../blocks/defaultProps.js";
+import { getBackgroundColorAttribute } from "../../blocks/defaultProps.js";
export const BackgroundColorExtension = Extension.create({
name: "blockBackgroundColor",
@@ -9,24 +9,7 @@ export const BackgroundColorExtension = Extension.create({
{
types: ["blockContainer", "tableCell", "tableHeader"],
attributes: {
- backgroundColor: {
- default: defaultProps.backgroundColor.default,
- parseHTML: (element) =>
- element.hasAttribute("data-background-color")
- ? element.getAttribute("data-background-color")
- : defaultProps.backgroundColor.default,
- renderHTML: (attributes) => {
- if (
- attributes.backgroundColor ===
- defaultProps.backgroundColor.default
- ) {
- return {};
- }
- return {
- "data-background-color": attributes.backgroundColor,
- };
- },
- },
+ backgroundColor: getBackgroundColorAttribute(),
},
},
];
diff --git a/packages/core/src/extensions/BackgroundColor/BackgroundColorMark.ts b/packages/core/src/extensions/BackgroundColor/BackgroundColorMark.ts
index 03c57dd4ac..1ef31cb1f4 100644
--- a/packages/core/src/extensions/BackgroundColor/BackgroundColorMark.ts
+++ b/packages/core/src/extensions/BackgroundColor/BackgroundColorMark.ts
@@ -1,4 +1,5 @@
import { Mark } from "@tiptap/core";
+import { getBackgroundColorAttribute } from "../../blocks/defaultProps.js";
import { createStyleSpecFromTipTapMark } from "../../schema/index.js";
const BackgroundColorMark = Mark.create({
@@ -6,13 +7,7 @@ const BackgroundColorMark = Mark.create({
addAttributes() {
return {
- stringValue: {
- default: undefined,
- parseHTML: (element) => element.getAttribute("data-background-color"),
- renderHTML: (attributes) => ({
- "data-background-color": attributes.stringValue,
- }),
- },
+ stringValue: getBackgroundColorAttribute("stringValue"),
};
},
@@ -25,10 +20,11 @@ const BackgroundColorMark = Mark.create({
return false;
}
- if (element.hasAttribute("data-background-color")) {
- return {
- stringValue: element.getAttribute("data-background-color"),
- };
+ if (
+ element.hasAttribute("data-background-color") ||
+ element.style.backgroundColor
+ ) {
+ return {};
}
return false;
diff --git a/packages/core/src/extensions/TextColor/TextColorExtension.ts b/packages/core/src/extensions/TextColor/TextColorExtension.ts
index 4060fea6d6..eddb96f9e7 100644
--- a/packages/core/src/extensions/TextColor/TextColorExtension.ts
+++ b/packages/core/src/extensions/TextColor/TextColorExtension.ts
@@ -1,5 +1,5 @@
import { Extension } from "@tiptap/core";
-import { defaultProps } from "../../blocks/defaultProps.js";
+import { getTextColorAttribute } from "../../blocks/defaultProps.js";
export const TextColorExtension = Extension.create({
name: "blockTextColor",
@@ -9,21 +9,7 @@ export const TextColorExtension = Extension.create({
{
types: ["blockContainer", "tableCell", "tableHeader"],
attributes: {
- textColor: {
- default: defaultProps.textColor.default,
- parseHTML: (element) =>
- element.hasAttribute("data-text-color")
- ? element.getAttribute("data-text-color")
- : defaultProps.textColor.default,
- renderHTML: (attributes) => {
- if (attributes.textColor === defaultProps.textColor.default) {
- return {};
- }
- return {
- "data-text-color": attributes.textColor,
- };
- },
- },
+ textColor: getTextColorAttribute(),
},
},
];
diff --git a/packages/core/src/extensions/TextColor/TextColorMark.ts b/packages/core/src/extensions/TextColor/TextColorMark.ts
index 2f5c314ba3..cc42f9a749 100644
--- a/packages/core/src/extensions/TextColor/TextColorMark.ts
+++ b/packages/core/src/extensions/TextColor/TextColorMark.ts
@@ -1,18 +1,14 @@
import { Mark } from "@tiptap/core";
+import { getTextColorAttribute } from "../../blocks/defaultProps.js";
import { createStyleSpecFromTipTapMark } from "../../schema/index.js";
const TextColorMark = Mark.create({
name: "textColor",
+ priority: 1000,
addAttributes() {
return {
- stringValue: {
- default: undefined,
- parseHTML: (element) => element.getAttribute("data-text-color"),
- renderHTML: (attributes) => ({
- "data-text-color": attributes.stringValue,
- }),
- },
+ stringValue: getTextColorAttribute("stringValue"),
};
},
@@ -25,8 +21,8 @@ const TextColorMark = Mark.create({
return false;
}
- if (element.hasAttribute("data-text-color")) {
- return { stringValue: element.getAttribute("data-text-color") };
+ if (element.hasAttribute("data-text-color") || element.style.color) {
+ return {};
}
return false;
diff --git a/packages/server-util/src/context/__snapshots__/ServerBlockNoteEditor.test.ts.snap b/packages/server-util/src/context/__snapshots__/ServerBlockNoteEditor.test.ts.snap
index 5bd969bfa8..ac3cc4b2c5 100644
--- a/packages/server-util/src/context/__snapshots__/ServerBlockNoteEditor.test.ts.snap
+++ b/packages/server-util/src/context/__snapshots__/ServerBlockNoteEditor.test.ts.snap
@@ -2,7 +2,7 @@
exports[`Test ServerBlockNoteEditor > converts to HTML (blocksToFullHTML) 1`] = `""`;
-exports[`Test ServerBlockNoteEditor > converts to and from HTML (blocksToHTMLLossy) 1`] = `"Heading 2
Paragraph
Caption"`;
+exports[`Test ServerBlockNoteEditor > converts to and from HTML (blocksToHTMLLossy) 1`] = `"Heading 2
Paragraph
Caption"`;
exports[`Test ServerBlockNoteEditor > converts to and from HTML (blocksToHTMLLossy) 2`] = `
[
@@ -26,12 +26,12 @@ exports[`Test ServerBlockNoteEditor > converts to and from HTML (blocksToHTMLLos
"type": "text",
},
],
- "id": "0",
+ "id": "1",
"props": {
- "backgroundColor": "default",
+ "backgroundColor": "blue",
"level": 2,
"textAlignment": "right",
- "textColor": "default",
+ "textColor": "yellow",
},
"type": "heading",
},
@@ -44,9 +44,9 @@ exports[`Test ServerBlockNoteEditor > converts to and from HTML (blocksToHTMLLos
"type": "text",
},
],
- "id": "1",
+ "id": "2",
"props": {
- "backgroundColor": "default",
+ "backgroundColor": "red",
"textAlignment": "left",
"textColor": "default",
},
@@ -61,7 +61,7 @@ exports[`Test ServerBlockNoteEditor > converts to and from HTML (blocksToHTMLLos
"type": "text",
},
],
- "id": "2",
+ "id": "3",
"props": {
"backgroundColor": "default",
"textAlignment": "left",
@@ -72,7 +72,7 @@ exports[`Test ServerBlockNoteEditor > converts to and from HTML (blocksToHTMLLos
{
"children": [],
"content": undefined,
- "id": "3",
+ "id": "4",
"props": {
"backgroundColor": "default",
"caption": "Caption",
@@ -86,43 +86,18 @@ exports[`Test ServerBlockNoteEditor > converts to and from HTML (blocksToHTMLLos
},
{
"children": [],
- "content": [
- {
- "content": [
- {
- "styles": {},
- "text": "Example",
- "type": "text",
- },
- ],
- "href": "exampleURL",
- "type": "link",
- },
- ],
- "id": "4",
- "props": {
- "backgroundColor": "default",
- "textAlignment": "left",
- "textColor": "default",
- },
- "type": "paragraph",
- },
- {
- "children": [],
- "content": [
- {
- "styles": {},
- "text": "Caption",
- "type": "text",
- },
- ],
+ "content": undefined,
"id": "5",
"props": {
"backgroundColor": "default",
+ "caption": "Caption",
+ "name": "Example",
+ "previewWidth": 256,
+ "showPreview": false,
"textAlignment": "left",
- "textColor": "default",
+ "url": "exampleURL",
},
- "type": "paragraph",
+ "type": "image",
},
]
`;
diff --git a/packages/xl-multi-column/src/test/conversions/__snapshots__/multi-column/undefined/external.html b/packages/xl-multi-column/src/test/conversions/__snapshots__/multi-column/undefined/external.html
index cbbcb6592b..b0d9e1665e 100644
--- a/packages/xl-multi-column/src/test/conversions/__snapshots__/multi-column/undefined/external.html
+++ b/packages/xl-multi-column/src/test/conversions/__snapshots__/multi-column/undefined/external.html
@@ -1 +1 @@
-Column Paragraph 0
Column Paragraph 1
Column Paragraph 2
Column Paragraph 3
\ No newline at end of file
+Column Paragraph 0
Column Paragraph 1
Column Paragraph 2
Column Paragraph 3
\ No newline at end of file
diff --git a/tests/src/unit/core/formatConversion/exportParseEquality/exportParseEqualityTestInstances.ts b/tests/src/unit/core/formatConversion/exportParseEquality/exportParseEqualityTestInstances.ts
index 63d3552df0..f8c886568b 100644
--- a/tests/src/unit/core/formatConversion/exportParseEquality/exportParseEqualityTestInstances.ts
+++ b/tests/src/unit/core/formatConversion/exportParseEquality/exportParseEqualityTestInstances.ts
@@ -21,3 +21,181 @@ export const exportParseEqualityTestInstancesBlockNoteHTML: TestInstance<
testCase,
executeTest: testExportParseEqualityBlockNoteHTML,
}));
+
+export const exportParseEqualityTestInstancesHTML: TestInstance<
+ ExportParseEqualityTestCase<
+ TestBlockSchema,
+ TestInlineContentSchema,
+ TestStyleSchema
+ >,
+ TestBlockSchema,
+ TestInlineContentSchema,
+ TestStyleSchema
+>[] = [
+ {
+ testCase: {
+ name: "schema/blocks",
+ content: [
+ {
+ type: "paragraph",
+ content: "Paragraph",
+ },
+ {
+ type: "heading",
+ content: "Heading",
+ },
+ {
+ type: "quote",
+ content: "Quote",
+ },
+ {
+ type: "bulletListItem",
+ content: "Bullet List Item",
+ },
+ {
+ type: "numberedListItem",
+ content: "Numbered List Item",
+ },
+ {
+ type: "checkListItem",
+ content: "Check List Item",
+ },
+ {
+ type: "codeBlock",
+ content: "Code",
+ },
+ {
+ type: "table",
+ content: {
+ type: "tableContent",
+ rows: [
+ {
+ cells: ["Table Cell", "Table Cell"],
+ },
+ {
+ cells: ["Table Cell", "Table Cell"],
+ },
+ ],
+ },
+ },
+ ],
+ },
+ executeTest: testExportParseEqualityHTML,
+ },
+ {
+ testCase: {
+ name: "schema/blockProps",
+ content: [
+ {
+ type: "paragraph",
+ content: "Paragraph",
+ props: {
+ textColor: "red",
+ backgroundColor: "blue",
+ textAlignment: "center",
+ },
+ },
+ {
+ type: "heading",
+ content: "Heading",
+ props: {
+ level: 2,
+ },
+ },
+ {
+ type: "checkListItem",
+ content: "Check List Item",
+ props: {
+ checked: true,
+ textColor: "red",
+ backgroundColor: "blue",
+ textAlignment: "center",
+ },
+ },
+ {
+ type: "codeBlock",
+ content: "Code",
+ props: { language: "javascript" },
+ },
+ ],
+ },
+ executeTest: testExportParseEqualityHTML,
+ },
+ {
+ testCase: {
+ name: "schema/inlineContent",
+ content: [
+ {
+ type: "paragraph",
+ content: [
+ {
+ type: "text",
+ text: "Text ",
+ styles: {},
+ },
+ {
+ type: "link",
+ content: "Link",
+ href: "https://example.com",
+ },
+ ],
+ },
+ ],
+ },
+ executeTest: testExportParseEqualityHTML,
+ },
+ {
+ testCase: {
+ name: "schema/styles",
+ content: [
+ {
+ type: "paragraph",
+ content: [
+ {
+ type: "text",
+ text: "T",
+ styles: {
+ bold: true,
+ italic: true,
+ underline: true,
+ strike: true,
+ // Code cannot be applied on top of other styles.
+ // code: true,
+ textColor: "red",
+ backgroundColor: "blue",
+ },
+ },
+ ],
+ },
+ ],
+ },
+ executeTest: testExportParseEqualityHTML,
+ },
+ // TODO: Tests failing
+ // {
+ // testCase: {
+ // name: "lists/nested",
+ // content: [
+ // {
+ // type: "bulletListItem",
+ // content: "List Item 1",
+ // children: [
+ // {
+ // type: "bulletListItem",
+ // content: "Nested List Item 1",
+ // },
+ // {
+ // type: "bulletListItem",
+ // content: "Nested List Item 2",
+ // },
+ // ],
+ // },
+ // {
+ // type: "bulletListItem",
+ // content: "List Item 2",
+ // },
+ // ],
+ // },
+ // executeTest: testExportParseEqualityHTML,
+ // },
+];
diff --git a/tests/src/unit/core/formatConversion/parse/__snapshots__/html/backgroundColorStyle.json b/tests/src/unit/core/formatConversion/parse/__snapshots__/html/backgroundColorStyle.json
new file mode 100644
index 0000000000..0f1dfc15e7
--- /dev/null
+++ b/tests/src/unit/core/formatConversion/parse/__snapshots__/html/backgroundColorStyle.json
@@ -0,0 +1,45 @@
+[
+ {
+ "children": [],
+ "content": [
+ {
+ "styles": {
+ "backgroundColor": "red",
+ },
+ "text": "Red Background",
+ "type": "text",
+ },
+ {
+ "styles": {},
+ "text": " ",
+ "type": "text",
+ },
+ {
+ "styles": {
+ "backgroundColor": "green",
+ },
+ "text": "Green Background",
+ "type": "text",
+ },
+ {
+ "styles": {},
+ "text": " ",
+ "type": "text",
+ },
+ {
+ "styles": {
+ "backgroundColor": "blue",
+ },
+ "text": "Blue Background",
+ "type": "text",
+ },
+ ],
+ "id": "1",
+ "props": {
+ "backgroundColor": "default",
+ "textAlignment": "left",
+ "textColor": "default",
+ },
+ "type": "paragraph",
+ },
+]
\ No newline at end of file
diff --git a/tests/src/unit/core/formatConversion/parse/__snapshots__/html/boldStyle.json b/tests/src/unit/core/formatConversion/parse/__snapshots__/html/boldStyle.json
new file mode 100644
index 0000000000..6a084ab50f
--- /dev/null
+++ b/tests/src/unit/core/formatConversion/parse/__snapshots__/html/boldStyle.json
@@ -0,0 +1,45 @@
+[
+ {
+ "children": [],
+ "content": [
+ {
+ "styles": {
+ "bold": true,
+ },
+ "text": "Bold",
+ "type": "text",
+ },
+ {
+ "styles": {},
+ "text": " ",
+ "type": "text",
+ },
+ {
+ "styles": {
+ "bold": true,
+ },
+ "text": "Bold",
+ "type": "text",
+ },
+ {
+ "styles": {},
+ "text": " ",
+ "type": "text",
+ },
+ {
+ "styles": {
+ "bold": true,
+ },
+ "text": "Bold",
+ "type": "text",
+ },
+ ],
+ "id": "1",
+ "props": {
+ "backgroundColor": "default",
+ "textAlignment": "left",
+ "textColor": "default",
+ },
+ "type": "paragraph",
+ },
+]
\ No newline at end of file
diff --git a/tests/src/unit/core/formatConversion/parse/__snapshots__/html/googleDocs.json b/tests/src/unit/core/formatConversion/parse/__snapshots__/html/googleDocs.json
index 5a7912dcd1..aeee039f19 100644
--- a/tests/src/unit/core/formatConversion/parse/__snapshots__/html/googleDocs.json
+++ b/tests/src/unit/core/formatConversion/parse/__snapshots__/html/googleDocs.json
@@ -5,6 +5,7 @@
{
"styles": {
"bold": true,
+ "textColor": "rgb(0, 0, 0)",
},
"text": "Heading 1",
"type": "text",
@@ -25,6 +26,7 @@
{
"styles": {
"bold": true,
+ "textColor": "rgb(0, 0, 0)",
},
"text": "Heading 2",
"type": "text",
@@ -45,6 +47,7 @@
{
"styles": {
"bold": true,
+ "textColor": "rgb(0, 0, 0)",
},
"text": "Heading 3",
"type": "text",
@@ -63,7 +66,9 @@
"children": [],
"content": [
{
- "styles": {},
+ "styles": {
+ "textColor": "rgb(0, 0, 0)",
+ },
"text": "Paragraph 1",
"type": "text",
},
@@ -80,7 +85,9 @@
"children": [],
"content": [
{
- "styles": {},
+ "styles": {
+ "textColor": "rgb(0, 0, 0)",
+ },
"text": "Paragraph 2",
"type": "text",
},
@@ -97,7 +104,9 @@
"children": [],
"content": [
{
- "styles": {},
+ "styles": {
+ "textColor": "rgb(0, 0, 0)",
+ },
"text": "Paragraph 3",
"type": "text",
},
@@ -114,7 +123,9 @@
"children": [],
"content": [
{
- "styles": {},
+ "styles": {
+ "textColor": "rgb(0, 0, 0)",
+ },
"text": "Paragraph With
Hard Break",
"type": "text",
@@ -134,36 +145,45 @@ Hard Break",
{
"styles": {
"bold": true,
+ "textColor": "rgb(0, 0, 0)",
},
"text": "Bold",
"type": "text",
},
{
- "styles": {},
+ "styles": {
+ "textColor": "rgb(0, 0, 0)",
+ },
"text": " ",
"type": "text",
},
{
"styles": {
"italic": true,
+ "textColor": "rgb(0, 0, 0)",
},
"text": "Italic",
"type": "text",
},
{
- "styles": {},
+ "styles": {
+ "textColor": "rgb(0, 0, 0)",
+ },
"text": " Underline ",
"type": "text",
},
{
"styles": {
"strike": true,
+ "textColor": "rgb(0, 0, 0)",
},
"text": "Strikethrough",
"type": "text",
},
{
- "styles": {},
+ "styles": {
+ "textColor": "rgb(0, 0, 0)",
+ },
"text": " ",
"type": "text",
},
@@ -172,6 +192,7 @@ Hard Break",
"bold": true,
"italic": true,
"strike": true,
+ "textColor": "rgb(255, 0, 0)",
},
"text": "All",
"type": "text",
@@ -194,7 +215,14 @@ Hard Break",
"content": [
{
"styles": {},
- "text": " Nested Numbered List Item 1",
+ "text": " ",
+ "type": "text",
+ },
+ {
+ "styles": {
+ "textColor": "rgb(0, 0, 0)",
+ },
+ "text": "Nested Numbered List Item 1",
"type": "text",
},
],
@@ -211,7 +239,14 @@ Hard Break",
"content": [
{
"styles": {},
- "text": " Nested Numbered List Item 2",
+ "text": " ",
+ "type": "text",
+ },
+ {
+ "styles": {
+ "textColor": "rgb(0, 0, 0)",
+ },
+ "text": "Nested Numbered List Item 2",
"type": "text",
},
],
@@ -227,7 +262,14 @@ Hard Break",
"content": [
{
"styles": {},
- "text": " Nested Bullet List Item 1",
+ "text": " ",
+ "type": "text",
+ },
+ {
+ "styles": {
+ "textColor": "rgb(0, 0, 0)",
+ },
+ "text": "Nested Bullet List Item 1",
"type": "text",
},
],
@@ -244,7 +286,14 @@ Hard Break",
"content": [
{
"styles": {},
- "text": " Nested Bullet List Item 2",
+ "text": " ",
+ "type": "text",
+ },
+ {
+ "styles": {
+ "textColor": "rgb(0, 0, 0)",
+ },
+ "text": "Nested Bullet List Item 2",
"type": "text",
},
],
@@ -260,7 +309,14 @@ Hard Break",
"content": [
{
"styles": {},
- "text": " Bullet List Item 1",
+ "text": " ",
+ "type": "text",
+ },
+ {
+ "styles": {
+ "textColor": "rgb(0, 0, 0)",
+ },
+ "text": "Bullet List Item 1",
"type": "text",
},
],
@@ -277,7 +333,14 @@ Hard Break",
"content": [
{
"styles": {},
- "text": " Bullet List Item 2",
+ "text": " ",
+ "type": "text",
+ },
+ {
+ "styles": {
+ "textColor": "rgb(0, 0, 0)",
+ },
+ "text": "Bullet List Item 2",
"type": "text",
},
],
@@ -294,7 +357,14 @@ Hard Break",
"content": [
{
"styles": {},
- "text": " Numbered List Item 1",
+ "text": " ",
+ "type": "text",
+ },
+ {
+ "styles": {
+ "textColor": "rgb(0, 0, 0)",
+ },
+ "text": "Numbered List Item 1",
"type": "text",
},
],
@@ -311,7 +381,14 @@ Hard Break",
"content": [
{
"styles": {},
- "text": " Numbered List Item 2",
+ "text": " ",
+ "type": "text",
+ },
+ {
+ "styles": {
+ "textColor": "rgb(0, 0, 0)",
+ },
+ "text": "Numbered List Item 2",
"type": "text",
},
],
@@ -372,7 +449,9 @@ Hard Break",
{
"content": [
{
- "styles": {},
+ "styles": {
+ "textColor": "rgb(0, 0, 0)",
+ },
"text": "Cell 1",
"type": "text",
},
@@ -389,7 +468,9 @@ Hard Break",
{
"content": [
{
- "styles": {},
+ "styles": {
+ "textColor": "rgb(0, 0, 0)",
+ },
"text": "Cell 2",
"type": "text",
},
@@ -406,7 +487,9 @@ Hard Break",
{
"content": [
{
- "styles": {},
+ "styles": {
+ "textColor": "rgb(0, 0, 0)",
+ },
"text": "Cell 3",
"type": "text",
},
@@ -427,7 +510,9 @@ Hard Break",
{
"content": [
{
- "styles": {},
+ "styles": {
+ "textColor": "rgb(0, 0, 0)",
+ },
"text": "Cell 4",
"type": "text",
},
@@ -444,7 +529,9 @@ Hard Break",
{
"content": [
{
- "styles": {},
+ "styles": {
+ "textColor": "rgb(0, 0, 0)",
+ },
"text": "Cell 5",
"type": "text",
},
@@ -461,7 +548,9 @@ Hard Break",
{
"content": [
{
- "styles": {},
+ "styles": {
+ "textColor": "rgb(0, 0, 0)",
+ },
"text": "Cell 6",
"type": "text",
},
@@ -482,7 +571,9 @@ Hard Break",
{
"content": [
{
- "styles": {},
+ "styles": {
+ "textColor": "rgb(0, 0, 0)",
+ },
"text": "Cell 7",
"type": "text",
},
@@ -499,7 +590,9 @@ Hard Break",
{
"content": [
{
- "styles": {},
+ "styles": {
+ "textColor": "rgb(0, 0, 0)",
+ },
"text": "Cell 8",
"type": "text",
},
@@ -516,7 +609,9 @@ Hard Break",
{
"content": [
{
- "styles": {},
+ "styles": {
+ "textColor": "rgb(0, 0, 0)",
+ },
"text": "Cell 9",
"type": "text",
},
@@ -545,7 +640,9 @@ Hard Break",
"children": [],
"content": [
{
- "styles": {},
+ "styles": {
+ "textColor": "rgb(0, 0, 0)",
+ },
"text": "Paragraph",
"type": "text",
},
diff --git a/tests/src/unit/core/formatConversion/parse/__snapshots__/html/imageWidth.json b/tests/src/unit/core/formatConversion/parse/__snapshots__/html/imageWidth.json
new file mode 100644
index 0000000000..61fe6080a0
--- /dev/null
+++ b/tests/src/unit/core/formatConversion/parse/__snapshots__/html/imageWidth.json
@@ -0,0 +1,17 @@
+[
+ {
+ "children": [],
+ "content": undefined,
+ "id": "1",
+ "props": {
+ "backgroundColor": "default",
+ "caption": "",
+ "name": "",
+ "previewWidth": 100,
+ "showPreview": true,
+ "textAlignment": "left",
+ "url": "exampleURL",
+ },
+ "type": "image",
+ },
+]
\ No newline at end of file
diff --git a/tests/src/unit/core/formatConversion/parse/__snapshots__/html/italicStyle.json b/tests/src/unit/core/formatConversion/parse/__snapshots__/html/italicStyle.json
new file mode 100644
index 0000000000..27d939a344
--- /dev/null
+++ b/tests/src/unit/core/formatConversion/parse/__snapshots__/html/italicStyle.json
@@ -0,0 +1,45 @@
+[
+ {
+ "children": [],
+ "content": [
+ {
+ "styles": {
+ "italic": true,
+ },
+ "text": "Italic",
+ "type": "text",
+ },
+ {
+ "styles": {},
+ "text": " ",
+ "type": "text",
+ },
+ {
+ "styles": {
+ "italic": true,
+ },
+ "text": "Italic",
+ "type": "text",
+ },
+ {
+ "styles": {},
+ "text": " ",
+ "type": "text",
+ },
+ {
+ "styles": {
+ "italic": true,
+ },
+ "text": "Italic",
+ "type": "text",
+ },
+ ],
+ "id": "1",
+ "props": {
+ "backgroundColor": "default",
+ "textAlignment": "left",
+ "textColor": "default",
+ },
+ "type": "paragraph",
+ },
+]
\ No newline at end of file
diff --git a/tests/src/unit/core/formatConversion/parse/__snapshots__/html/orderedListStart.json b/tests/src/unit/core/formatConversion/parse/__snapshots__/html/orderedListStart.json
new file mode 100644
index 0000000000..3532f949a8
--- /dev/null
+++ b/tests/src/unit/core/formatConversion/parse/__snapshots__/html/orderedListStart.json
@@ -0,0 +1,54 @@
+[
+ {
+ "children": [],
+ "content": [
+ {
+ "styles": {},
+ "text": "List Item 2",
+ "type": "text",
+ },
+ ],
+ "id": "1",
+ "props": {
+ "backgroundColor": "default",
+ "start": 2,
+ "textAlignment": "left",
+ "textColor": "default",
+ },
+ "type": "numberedListItem",
+ },
+ {
+ "children": [],
+ "content": [
+ {
+ "styles": {},
+ "text": "List Item 3",
+ "type": "text",
+ },
+ ],
+ "id": "2",
+ "props": {
+ "backgroundColor": "default",
+ "textAlignment": "left",
+ "textColor": "default",
+ },
+ "type": "numberedListItem",
+ },
+ {
+ "children": [],
+ "content": [
+ {
+ "styles": {},
+ "text": "List Item 4",
+ "type": "text",
+ },
+ ],
+ "id": "3",
+ "props": {
+ "backgroundColor": "default",
+ "textAlignment": "left",
+ "textColor": "default",
+ },
+ "type": "numberedListItem",
+ },
+]
\ No newline at end of file
diff --git a/tests/src/unit/core/formatConversion/parse/__snapshots__/html/strikeStyle.json b/tests/src/unit/core/formatConversion/parse/__snapshots__/html/strikeStyle.json
new file mode 100644
index 0000000000..cedb32736f
--- /dev/null
+++ b/tests/src/unit/core/formatConversion/parse/__snapshots__/html/strikeStyle.json
@@ -0,0 +1,57 @@
+[
+ {
+ "children": [],
+ "content": [
+ {
+ "styles": {
+ "strike": true,
+ },
+ "text": "Strike",
+ "type": "text",
+ },
+ {
+ "styles": {},
+ "text": " ",
+ "type": "text",
+ },
+ {
+ "styles": {
+ "strike": true,
+ },
+ "text": "Strike",
+ "type": "text",
+ },
+ {
+ "styles": {},
+ "text": " ",
+ "type": "text",
+ },
+ {
+ "styles": {
+ "strike": true,
+ },
+ "text": "Strike",
+ "type": "text",
+ },
+ {
+ "styles": {},
+ "text": " ",
+ "type": "text",
+ },
+ {
+ "styles": {
+ "strike": true,
+ },
+ "text": "Strike",
+ "type": "text",
+ },
+ ],
+ "id": "1",
+ "props": {
+ "backgroundColor": "default",
+ "textAlignment": "left",
+ "textColor": "default",
+ },
+ "type": "paragraph",
+ },
+]
\ No newline at end of file
diff --git a/tests/src/unit/core/formatConversion/parse/__snapshots__/html/textColorStyle.json b/tests/src/unit/core/formatConversion/parse/__snapshots__/html/textColorStyle.json
new file mode 100644
index 0000000000..faeeee5277
--- /dev/null
+++ b/tests/src/unit/core/formatConversion/parse/__snapshots__/html/textColorStyle.json
@@ -0,0 +1,45 @@
+[
+ {
+ "children": [],
+ "content": [
+ {
+ "styles": {
+ "textColor": "red",
+ },
+ "text": "Red Text",
+ "type": "text",
+ },
+ {
+ "styles": {},
+ "text": " ",
+ "type": "text",
+ },
+ {
+ "styles": {
+ "textColor": "green",
+ },
+ "text": "Green Text",
+ "type": "text",
+ },
+ {
+ "styles": {},
+ "text": " ",
+ "type": "text",
+ },
+ {
+ "styles": {
+ "textColor": "blue",
+ },
+ "text": "Blue Text",
+ "type": "text",
+ },
+ ],
+ "id": "1",
+ "props": {
+ "backgroundColor": "default",
+ "textAlignment": "left",
+ "textColor": "default",
+ },
+ "type": "paragraph",
+ },
+]
\ No newline at end of file
diff --git a/tests/src/unit/core/formatConversion/parse/__snapshots__/html/underlineStyle.json b/tests/src/unit/core/formatConversion/parse/__snapshots__/html/underlineStyle.json
new file mode 100644
index 0000000000..22472ff797
--- /dev/null
+++ b/tests/src/unit/core/formatConversion/parse/__snapshots__/html/underlineStyle.json
@@ -0,0 +1,33 @@
+[
+ {
+ "children": [],
+ "content": [
+ {
+ "styles": {
+ "underline": true,
+ },
+ "text": "Underline",
+ "type": "text",
+ },
+ {
+ "styles": {},
+ "text": " ",
+ "type": "text",
+ },
+ {
+ "styles": {
+ "underline": true,
+ },
+ "text": "Underline",
+ "type": "text",
+ },
+ ],
+ "id": "1",
+ "props": {
+ "backgroundColor": "default",
+ "textAlignment": "left",
+ "textColor": "default",
+ },
+ "type": "paragraph",
+ },
+]
\ No newline at end of file
diff --git a/tests/src/unit/core/formatConversion/parse/parseTestInstances.ts b/tests/src/unit/core/formatConversion/parse/parseTestInstances.ts
index 1953d1ca75..2254316478 100644
--- a/tests/src/unit/core/formatConversion/parse/parseTestInstances.ts
+++ b/tests/src/unit/core/formatConversion/parse/parseTestInstances.ts
@@ -350,57 +350,57 @@ export const parseTestInstancesHTML: TestInstance<
testCase: {
name: "notion",
content: `Heading 1
-Heading 2
-Heading 3
-Paragraph 1
-Nested Paragraph 1
-Nested Paragraph 2
-Paragraph
-With Hard Break
-Bold Italic Underline Strikethrough All
-
-- Bullet List Item 1
-
-- Nested Bullet List Item 1
-
-- Nested Numbered List Item 1
-- Nested Numbered List Item 2
-
-
-- Nested Bullet List Item 2
-
-
-- Bullet List Item 2
-
-
-- Numbered List Item 1
-- Numbered List Item 2
-
-Background Color Paragraph
-!https://www.pulsecarshalton.co.uk/wp-content/uploads/2016/08/jk-placeholder-image.jpg
-
-
-
-Cell 1 |
-Cell 2 |
-Cell 3 |
-
-
-
-
-Cell 4 |
-Cell 5 |
-Cell 6 |
-
-
-Cell 7 |
-Cell 8 |
-Cell 9 |
-
-
-
-Paragraph
-`,
+ Heading 2
+ Heading 3
+ Paragraph 1
+ Nested Paragraph 1
+ Nested Paragraph 2
+ Paragraph
+ With Hard Break
+ Bold Italic Underline Strikethrough All
+
+ - Bullet List Item 1
+
+ - Nested Bullet List Item 1
+
+ - Nested Numbered List Item 1
+ - Nested Numbered List Item 2
+
+
+ - Nested Bullet List Item 2
+
+
+ - Bullet List Item 2
+
+
+ - Numbered List Item 1
+ - Numbered List Item 2
+
+ Background Color Paragraph
+ !https://www.pulsecarshalton.co.uk/wp-content/uploads/2016/08/jk-placeholder-image.jpg
+
+
+
+ Cell 1 |
+ Cell 2 |
+ Cell 3 |
+
+
+
+
+ Cell 4 |
+ Cell 5 |
+ Cell 6 |
+
+
+ Cell 7 |
+ Cell 8 |
+ Cell 9 |
+
+
+
+ Paragraph
+ `,
},
executeTest: testParseHTML,
},
@@ -408,97 +408,97 @@ With Hard Break
testCase: {
name: "googleDocs",
content: `
-
-
-Heading 1
-Heading 2
-Heading 3
-Paragraph 1
-Paragraph 2
-Paragraph 3
-Paragraph With
Hard Break
-Bold Italic Underline Strikethrough All
-
--
-
Bullet List Item 1
-
-
--
-
Bullet List Item 2
-
-
-
--
-
Numbered List Item 1
-
--
-
Numbered List Item 2
-
-
-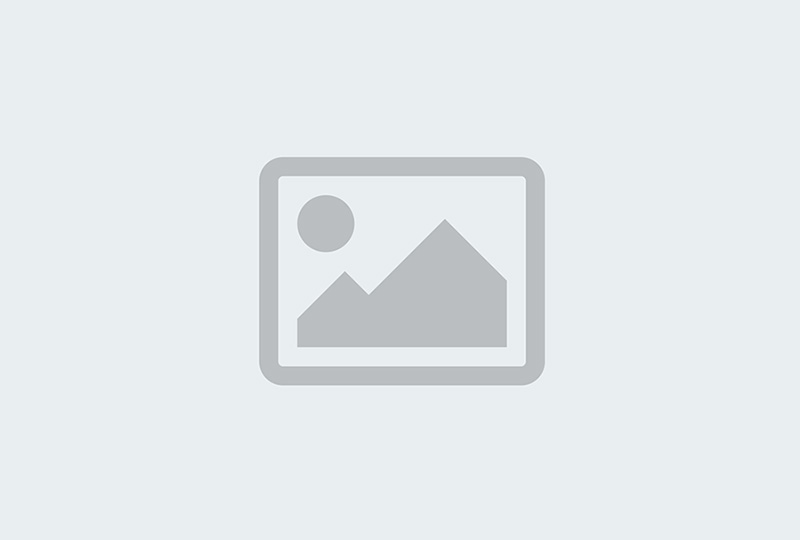
-
-
-
-
-
-
-
-
-
-
-
- Cell 1
- |
-
- Cell 2
- |
-
- Cell 3
- |
-
-
-
- Cell 4
- |
-
- Cell 5
- |
-
- Cell 6
- |
-
-
-
- Cell 7
- |
-
- Cell 8
- |
-
- Cell 9
- |
-
-
-
-
-Paragraph
-
-
`,
+
+
+ Heading 1
+ Heading 2
+ Heading 3
+ Paragraph 1
+ Paragraph 2
+ Paragraph 3
+ Paragraph With
Hard Break
+ Bold Italic Underline Strikethrough All
+
+ -
+
Bullet List Item 1
+
+
+ -
+
Bullet List Item 2
+
+
+
+ -
+
Numbered List Item 1
+
+ -
+
Numbered List Item 2
+
+
+ 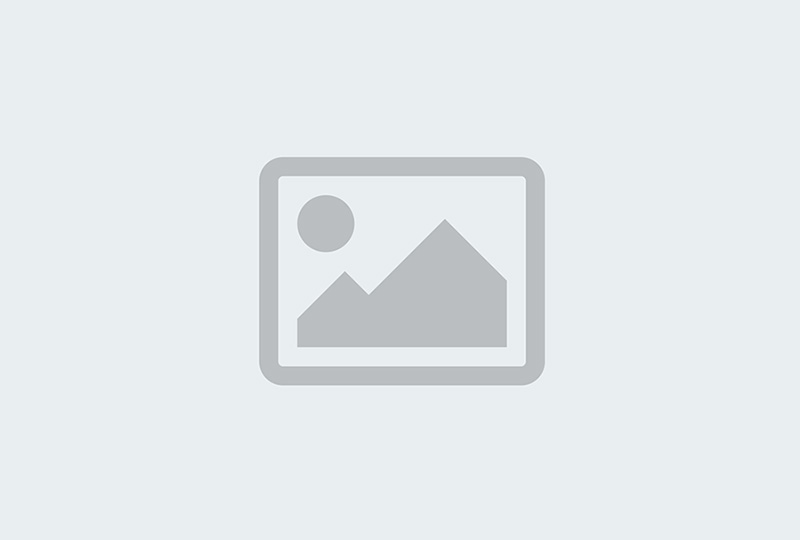
+
+
+
+
+
+
+
+
+
+
+
+ Cell 1
+ |
+
+ Cell 2
+ |
+
+ Cell 3
+ |
+
+
+
+ Cell 4
+ |
+
+ Cell 5
+ |
+
+ Cell 6
+ |
+
+
+
+ Cell 7
+ |
+
+ Cell 8
+ |
+
+ Cell 9
+ |
+
+
+
+
+ Paragraph
+
+
`,
},
executeTest: testParseHTML,
},
@@ -506,12 +506,98 @@ With Hard Break
testCase: {
name: "codeBlocks",
content: `console.log("Should default to JS")
-console.log("Should parse TS from data-language")
-print("Should parse Python from language- class")
-console.log("Should prioritize TS from data-language over language- class")
`,
+ console.log("Should parse TS from data-language")
+ print("Should parse Python from language- class")
+ console.log("Should prioritize TS from data-language over language- class")
`,
+ },
+ executeTest: testParseHTML,
+ },
+ {
+ testCase: {
+ name: "boldStyle",
+ content: `Bold Bold Bold
`,
+ },
+ executeTest: testParseHTML,
+ },
+ {
+ testCase: {
+ name: "italicStyle",
+ content: `Italic Italic Italic
`,
+ },
+ executeTest: testParseHTML,
+ },
+ {
+ testCase: {
+ name: "underlineStyle",
+ content: `Underline Underline
`,
+ },
+ executeTest: testParseHTML,
+ },
+ {
+ testCase: {
+ name: "strikeStyle",
+ content: `Strike Strike Strike Strike
`,
+ },
+ executeTest: testParseHTML,
+ },
+ {
+ testCase: {
+ name: "textColorStyle",
+ content: `Red Text Green Text Blue Text
`,
+ },
+ executeTest: testParseHTML,
+ },
+ {
+ testCase: {
+ name: "backgroundColorStyle",
+ content: `Red Background Green Background Blue Background
`,
+ },
+ executeTest: testParseHTML,
+ },
+ {
+ testCase: {
+ name: "orderedListStart",
+ content: `
+ - List Item 2
+ - List Item 3
+ - List Item 4
+
`,
+ },
+ executeTest: testParseHTML,
+ },
+ {
+ testCase: {
+ name: "imageWidth",
+ content: `
`,
},
executeTest: testParseHTML,
},
+ // TODO: Tests failing
+ // {
+ // testCase: {
+ // name: "textAlignmentProp",
+ // content: `Text Align Center
`,
+ // },
+ // executeTest: testParseHTML,
+ // },
+ // {
+ // testCase: {
+ // name: "textColorProp",
+ // content: `Red Paragraph
+ // Green Paragraph
+ // Blue Paragraph
`,
+ // },
+ // executeTest: testParseHTML,
+ // },
+ // {
+ // testCase: {
+ // name: "backgroundColorProp",
+ // content: `Red Background
+ // Green Background
+ // Blue Background
`,
+ // },
+ // executeTest: testParseHTML,
+ // },
];
export const parseTestInstancesMarkdown: TestInstance<