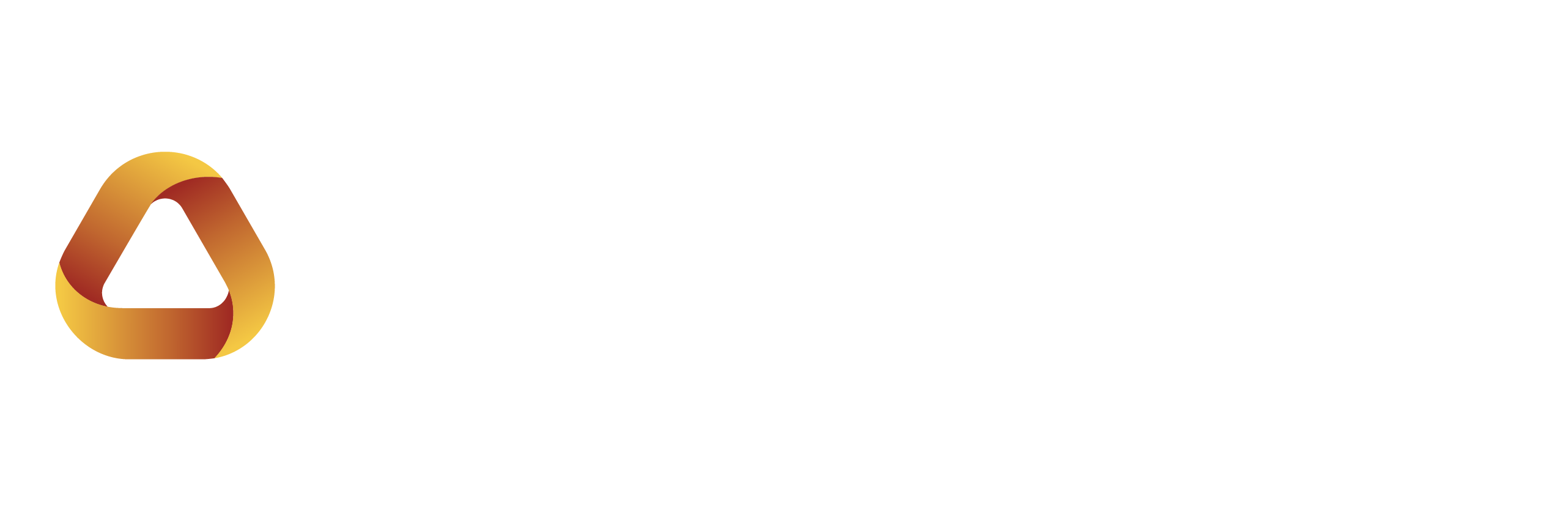
The DCAP Portal Contract is user-facing, and seamlessly submits DCAP quotes or (SNARK proofs) on the user's behalf to the Automata DCAP attestation contract.
Upon successful verification of a quote, the Portal performs a callback to the user contract with the provided calldata.
To begin building with DCAP Portal, the following dependencies must be installed on your machine:
- Foundry
- pnpm / npm
sequenceDiagram
autonumber
participant U as User
participant P as Portal
participant A as DCAP Attestation
participant C as User Contract
note over U: Generates DCAP Quote
U->>+P: Sends DCAP Quote
P->>A: Verifies DCAP Quote
alt Verification Passed
P->>+C: Callback
note over C: Checks whether function call is coming from the DCAP Portal contract address
note over C: Extracts the Attestation Output Data
C->>-P: Done
P->>U: Done
else
P->>-U: error VERIFICATION_FAILED()
end
- The user generates an Intel DCAP Quote, which they can either:
(a) Sends it directly to the Portal contract, via
verifyAndAttestOnChain()
or (b) Performs quote verificationn with zkVM Program executed either via RiscZero or SP1, a SNARK proof and the execution output are then sent to the contract viaverifyAndAttestWithZKProof
.
Regardless of which method the user invokes, they must specify the Callback
parameters.
-
The Portal forwards either (a) the DCAP quote for full on-chain verification or (b) SNARK proofs and the execution output to the Automata DCAP Attestation contract. Upon successful verification, the Attestation Output is returned to the Portal.
-
The Portal performs a callback on the user contract with calldata provided in the
Callback
parameters. -
The user contract first checks that a function call is sent by the Portal contract. Once confirmed the legitimacy of the quote attestation, it has the option to extract the quote report data from the Attestation Output.
-
The Portal returns SUCCESS.
-
The Portal throws a VERIFICATION_FAILED error for invalid quotes.
This section provides step-by-step instructions for integrating with DCAP Portal to your contract. See VerifiedCounter for an example.
1. Import DcapLibCallback.sol
import "@dcap-portal/lib/DcapLibCallback.sol"
2. Extend to your contract
contract VerifiedCounter is DcapLibCallback {
constructor(address _dcapPortalAddress) {
// Initial the DcapLibCallback
__DcapLibCallbackInit(_dcapPortalAddress);
}
}
3. Add your function and restrict the caller
contract VerifiedCounter is DcapLibCallback {
//...
event AttestationReportUserData(bytes);
function setNumber(uint256 newNumber) public fromDcapPortal {
number = newNumber;
// extract data from the output:
// * https://github.com/automata-network/automata-dcap-attestation/blob/2d8b6b3dd35643081fa7fd98bbb1323a0601b2bb/contracts/bases/QuoteVerifierBase.sol#L143
bytes memory attestationOutput = _attestationOutput();
// get the user data from the attestation report
emit AttestationReportUserData(_attestationReportUserData());
}
}
The fromDcapPortal()
modifier ensures that the number
value can only be changed after successful verification of the DCAP quote.
There are stricter modifiers available as well, that you may either require:
- A specific caller to the
DcapPortal
withfromDcapPortalAndSender()
OR
- A specific
tx.origin
withfromDcapPortalAndOrigin()