@@ -138,7 +138,7 @@ Let's start with a couple of "_familiar_" _generic_ functions
138
138
139
139
It's _ essential_ to ask: "_ Where do I ** start** (my ** TDD** quest)?_ " <br />
140
140
The answer is: create ** two** new files:
141
- ` examples/todo-list /elmish.js` and ` test/elmish.test.js `
141
+ ` lib /elmish.js` and ` test/elmish.test.js `
142
142
143
143
144
144
### Test Setup
@@ -152,9 +152,9 @@ const test = require('tape'); // https://github.com/dwyl/learn-tape
152
152
const fs = require (' fs' ); // to read html files (see below)
153
153
const path = require (' path' ); // so we can open files cross-platform
154
154
const html = fs .readFileSync (path .resolve (__dirname ,
155
- ' ../examples/todo-list/ index.html' )); // sample HTML file to initialise JSDOM.
155
+ ' ../index.html' )); // sample HTML file to initialise JSDOM.
156
156
require (' jsdom-global' )(html); // https://github.com/rstacruz/jsdom-global
157
- const elmish = require (' ../examples/todo-list /elmish.js' ); // functions to test
157
+ const elmish = require (' ../lib /elmish.js' ); // functions to test
158
158
const id = ' test-app' ; // all tests use 'test-app' as root element
159
159
```
160
160
@@ -184,7 +184,7 @@ it is used to erase the DOM before re-rendering the app.
184
184
185
185
Following "** _ Document(ation)_ Driven Development** ",
186
186
we create a ** ` JSDOC ` ** comment block
187
- in the ` examples/todo-list /elmish.js` file
187
+ in the ` lib /elmish.js` file
188
188
with _ just_ the function description:
189
189
190
190
``` js
@@ -259,7 +259,7 @@ You should see the following:
259
259
#### ` empty ` Function _ Implementation_
260
260
261
261
Now that we have the ** test** for our ` empty ` function written,
262
- we can add the ` empty ` function to ` examples/todo-list /elmish.js` :
262
+ we can add the ` empty ` function to ` lib /elmish.js` :
263
263
``` js
264
264
/**
265
265
* `empty` deletes all the DOM elements from within a specific "root" element.
@@ -281,7 +281,7 @@ function empty(node) {
281
281
282
282
Adding the function to the ` elmish.js ` file is a good _ start_ ,
283
283
but we need to *** ` export ` *** it to be able to _ invoke_ it in our test. <br />
284
- Add the following code at the end of ` examples/todo-list /elmish.js` :
284
+ Add the following code at the end of ` lib /elmish.js` :
285
285
286
286
``` js
287
287
/* module.exports is needed to run the functions using Node.js for testing! */
@@ -316,7 +316,7 @@ it "mounts" ("_renders_") the App in the "root" DOM element.
316
316
It also tells our app to "re-render"
317
317
when a ` signal ` with an ` action ` is received.
318
318
319
- In ` examples/todo-list /elmish.js` add the following ` JSDOC ` comment:
319
+ In ` lib /elmish.js` add the following ` JSDOC ` comment:
320
320
``` js
321
321
/**
322
322
* `mount` mounts the app in the "root" DOM Element.
@@ -333,7 +333,7 @@ In the `test/elmish.test.js` file, append the following code:
333
333
``` js
334
334
// use view and update from counter-reset example
335
335
// to invoke elmish.mount() function and confirm it is generic!
336
- const { view , update } = require (' ../examples/counter-reset /counter.js' );
336
+ const { view , update } = require (' ./counter.js' );
337
337
338
338
test (' elmish.mount app expect state to be Zero' , function (t ) {
339
339
const root = document .getElementById (id);
@@ -360,7 +360,7 @@ see:_ `test/counter-reset.test.js`
360
360
#### ` mount ` Function _ Implementation_
361
361
362
362
Add the following code to the ` mount ` function body to make the test _ pass_
363
- in ` examples/todo-list /elmish.js` :
363
+ in ` lib /elmish.js` :
364
364
``` js
365
365
/**
366
366
* `mount` mounts the app in the "root" DOM Element.
@@ -670,7 +670,7 @@ by writing the `add_attributes` function. <br />
670
670
(_ don't forget to_ ` export ` _ the function at the bottom of the file_ ).
671
671
672
672
If you get "stuck", checkout the _ complete_ example:
673
- [ /examples/todo-list /elmish.js] ( https://github.com/dwyl/learn-elm-architecture-in-javascript/tree/master/examples/todo-list/elmish.js )
673
+ [ /lib /elmish.js] ( https://github.com/dwyl/learn-elm-architecture-in-javascript/tree/master/examples/todo-list/elmish.js )
674
674
675
675
> ** Note 0** : we have "_ seen_ " the code _ before_ in the ` counter ` example:
676
676
> [ counter.js#L51] ( https://github.com/dwyl/learn-elm-architecture-in-javascript/blob/814467e81b1b9739da74378455bd12721b096ebd/examples/counter-reset/counter.js#L51 ) <br />
@@ -735,7 +735,7 @@ and make this test _pass_:
735
735
![ image] ( https://user-images.githubusercontent.com/194400/43416921-8506baaa-9431-11e8-9585-814e704a694d.png )
736
736
737
737
If you get "stuck", checkout the _ complete_ example:
738
- [ /examples/todo-list /elmish.js] ( https://github.com/dwyl/learn-elm-architecture-in-javascript/tree/master/examples/todo-list/elmish.js )
738
+ [ /lib /elmish.js] ( https://github.com/dwyl/learn-elm-architecture-in-javascript/tree/master/examples/todo-list/elmish.js )
739
739
740
740
<br />
741
741
@@ -1042,7 +1042,7 @@ test.only('elmish.add_attributes apply style="display: block;"', function (t) {
1042
1042
Write the "case" in to make this test _ pass_ in ` elmish.js ` .
1043
1043
1044
1044
If you get "stuck", checkout:
1045
- https://github.com/dwyl/learn- elm-architecture-in-javascript/tree /master/examples/todo-list /elmish.js
1045
+ https://github.com/dwyl/todomvc-vanilla-javascript- elm-architecture-example/blob /master/lib /elmish.js
1046
1046
1047
1047
<br />
1048
1048
@@ -1177,7 +1177,8 @@ It _should_ be the _easiest_ one so far.
1177
1177
Don't forget to remove the ` .only ` from the test, once you finish.
1178
1178
1179
1179
If you get "stuck", checkout:
1180
- https://github.com/dwyl/learn-elm-architecture-in-javascript/tree/master/examples/todo-list/elmish.js <br />
1180
+ [ ` lib/elmish.js ` ] ( https://github.com/dwyl/todomvc-vanilla-javascript-elm-architecture-example/blob/master/lib/elmish.js )
1181
+ <br />
1181
1182
1182
1183
1183
1184
### ` <section> ` HTML Element
@@ -1251,10 +1252,11 @@ Attempt to create the `section` function
1251
1252
using the ` add_attributes ` and ` append_childnodes ` "helper" functions.
1252
1253
1253
1254
If you get "stuck", checkout:
1254
- https://github.com/dwyl/learn-elm-architecture-in-javascript/tree/master/examples/todo-list/elmish.js <br />
1255
+ [ ` lib/elmish.js ` ] ( https://github.com/dwyl/todomvc-vanilla-javascript-elm-architecture-example/blob/master/lib/elmish.js )
1256
+ <br />
1255
1257
> _ ** Note** : in our "solution" we created a "helper" function
1256
1258
called ` create_element ` to "DRY" the HTML element creation code;
1257
- this is a * recommended** "best practice"._
1259
+ this is a * recommended** "best practice" improves maintainability ._
1258
1260
1259
1261
The ` JSDOC ` comment for our ` create_element ` function is:
1260
1262
``` js
@@ -1341,7 +1343,7 @@ but has only one argument and invokes a native method.
1341
1343
1342
1344
If you get stuck trying to make this test pass,
1343
1345
refer to the completed code:
1344
- [ /examples/todo-list /elmish.js] ( https://github.com/dwyl/learn-elm-architecture-in-javascript/tree/master/examples/todo-list/elmish.js )
1346
+ [ /lib /elmish.js] ( https://github.com/dwyl/learn-elm-architecture-in-javascript/tree/master/examples/todo-list/elmish.js )
1345
1347
1346
1348
1347
1349
#### Create the "main" ` view ` functions
@@ -1420,7 +1422,7 @@ Just make the tests pass and try to keep your code _maintainable_.
1420
1422
1421
1423
Again, if you get stuck trying to make this test pass,
1422
1424
refer to the completed code:
1423
- [ /examples/todo-list /elmish.js] ( https://github.com/dwyl/learn-elm-architecture-in-javascript/tree/master/examples/todo-list/elmish.js )
1425
+ [ /lib /elmish.js] ( https://github.com/dwyl/learn-elm-architecture-in-javascript/tree/master/examples/todo-list/elmish.js )
1424
1426
1425
1427
1426
1428
#### Create the ` <footer> ` view functions
@@ -1493,7 +1495,7 @@ to `elmish.js` and `export` them so the test will pass.
1493
1495
1494
1496
if you get stuck trying to make this test pass,
1495
1497
refer to the completed code:
1496
- [ /examples/todo-list /elmish.js] ( https://github.com/dwyl/learn-elm-architecture-in-javascript/tree/master/examples/todo-list/elmish.js )
1498
+ [ /lib /elmish.js] ( https://github.com/dwyl/learn-elm-architecture-in-javascript/tree/master/examples/todo-list/elmish.js )
1497
1499
1498
1500
<br />
1499
1501
@@ -1617,7 +1619,7 @@ Try and figure it out for yourself before checking a solution.
1617
1619
1618
1620
** ` if ` ** you get stuck trying to make this test pass,
1619
1621
refer to the completed code:
1620
- [ /examples/todo-list /elmish.js] ( https://github.com/dwyl/learn-elm-architecture-in-javascript/tree/master/examples/todo-list/elmish.js )
1622
+ [ /lib /elmish.js] ( https://github.com/dwyl/learn-elm-architecture-in-javascript/tree/master/examples/todo-list/elmish.js )
1621
1623
1622
1624
1623
1625
> _ ** Note** : do not "worry" about how to render the "right" content on the "page"
@@ -1738,7 +1740,7 @@ console.log('localStorage (polyfil) hello', localStorage.getItem('hello'));
1738
1740
// to confirm that our elmish.mount localStorage works and is "generic".
1739
1741
1740
1742
test .only (' elmish.mount sets model in localStorage' , function (t ) {
1741
- const { view , update } = require (' ../examples/counter-reset /counter.js' );
1743
+ const { view , update } = require (' ./counter.js' );
1742
1744
1743
1745
const root = document .getElementById (id);
1744
1746
elmish .mount (7 , update, view, id);
@@ -1793,7 +1795,7 @@ to make the test pass.
1793
1795
1794
1796
** ` if ` ** you get stuck trying to make this test pass,
1795
1797
refer to the completed code:
1796
- [ /examples/todo-list /elmish.js] ( https://github.com/dwyl/learn-elm-architecture-in-javascript/tree/master/examples/todo-list/elmish.js )
1798
+ [ /lib /elmish.js] ( https://github.com/dwyl/learn-elm-architecture-in-javascript/tree/master/examples/todo-list/elmish.js )
1797
1799
1798
1800
<br />
1799
1801
@@ -1896,26 +1898,27 @@ Background reading: https://webaim.org/techniques/keyboard
1896
1898
1897
1899
#### Baseline Example Code _ Without_ Subscription
1898
1900
1899
- Let's start by making a "copy" of the code in ` /examples/counter-reset ` :
1901
+ Let's start by making a "copy" of the code in
1902
+ [ ` /examples/counter-reset ` ] ( https://github.com/dwyl/learn-elm-architecture-in-javascript/tree/master/examples/counter-reset ) :
1903
+
1900
1904
``` sh
1901
- mkdir examples/counter-reset-keyboard
1902
- cp examples/counter-reset/* examples/counter-reset-keyboard/
1905
+ cp test/counter.js test/counter-reset-keyboard.js
1903
1906
```
1904
1907
1905
1908
_ First step_ is to _ re-factor_ the code in
1906
- ` examples /counter-reset-keyboard/counter .js`
1909
+ ` test /counter-reset-keyboard.js`
1907
1910
to use the "DOM" functions we've been creating for ` Elm ` (_ ish_ ).
1908
1911
This will _ simplify_ the ` counter.js ` down to the _ bare minimum_ .
1909
1912
1910
- In your ` examples /counter-reset-keyboard/counter .js` file,
1913
+ In your ` test /counter-reset-keyboard.js` file,
1911
1914
type the following code:
1912
1915
1913
1916
``` js
1914
1917
/* if require is available, it means we are in Node.js Land i.e. testing!
1915
1918
in the broweser, the "elmish" DOM functions are loaded in a <script> tag */
1916
1919
/* istanbul ignore next */
1917
1920
if (typeof require !== ' undefined' && this .window !== this ) {
1918
- var { button, div, empty, h1, mount, text } = require (' ./elmish.js' );
1921
+ var { button, div, empty, h1, mount, text } = require (' ../lib /elmish.js' );
1919
1922
}
1920
1923
1921
1924
function update (action , model ) { // Update function takes the current state
@@ -1945,20 +1948,20 @@ if (typeof module !== 'undefined' && module.exports) {
1945
1948
}
1946
1949
}
1947
1950
```
1948
-
1951
+ <!--
1949
1952
Without _touching_ the code/tests
1950
- in ** ` examples/counter-reset-keyboard/test.js ` ** ,
1953
+ in **`test/counter-reset-keyboard.js`**,
1954
+
1951
1955
You should just be able to _run_ the "liveserver" on your `localhost`:
1952
1956
1953
1957
```sh
1954
- npm start
1958
+ npm run dev
1955
1959
```
1956
-
1957
1960
and when you open: http://127.0.0.1:8000/examples/counter-reset-keyboard
1958
1961
1959
1962
should see the Qunit (Broweser) Tests _passing_:
1960
1963
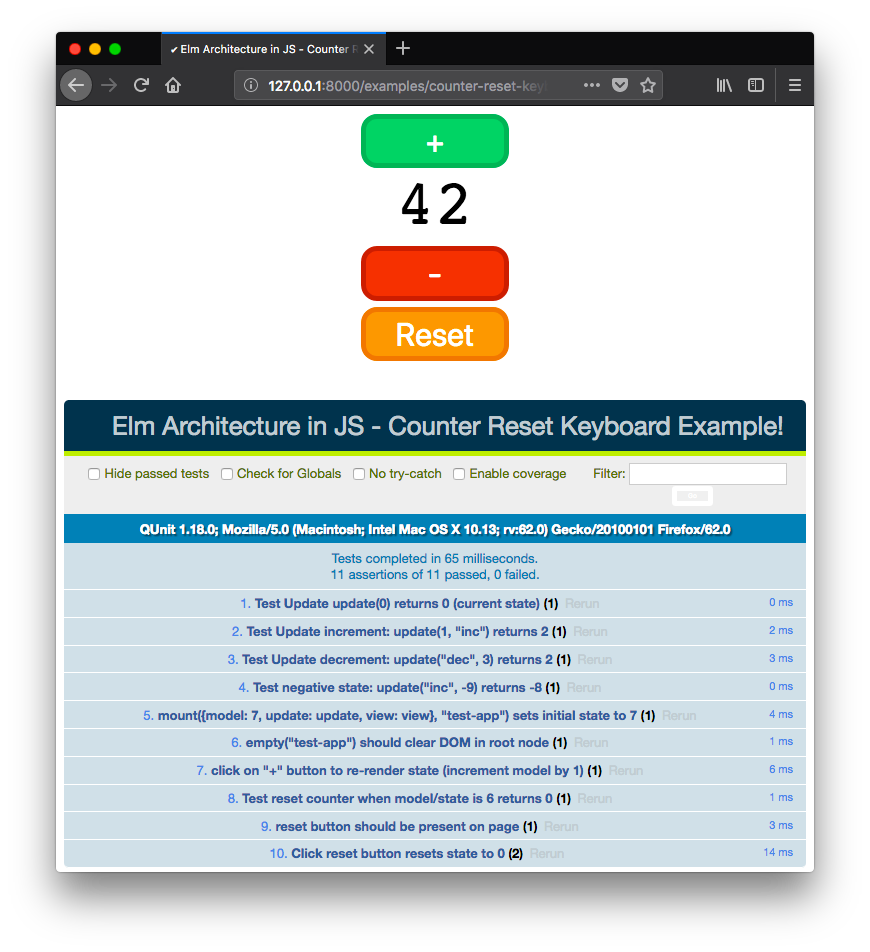
1961
-
1964
+ -->
1962
1965
1963
1966
#### How do We _ Test_ for Subscription Events?
1964
1967
@@ -2003,7 +2006,7 @@ It's "OK" to "take a peek" at the sample code:
2003
2006
[ ** ` examples/counter-reset-keyboard/counter.js ` ** ] ( https://github.com/dwyl/learn-elm-architecture-in-javascript/pull/45/files#diff-97353eabc55df91dbb3f96ba5a000a1aR26 )
2004
2007
2005
2008
Once you add the ** ` subscriptions ` ** function to
2006
- ** ` examples /counter-reset-keyboard/counter .js` ** ,
2009
+ ** ` test /counter-reset-keyboard.js` ** ,
2007
2010
Your tests should pass:
2008
2011
2009
2012
![ counter-reset-keyboard-subscriptions-tests-passing] ( https://user-images.githubusercontent.com/194400/43981911-b6413dda-9ceb-11e8-8514-44fc1f88c3fe.png )
@@ -2021,17 +2024,7 @@ for our TodoMVC App!
2021
2024
2022
2025
2023
2026
2024
-
2025
-
2026
-
2027
-
2028
-
2029
-
2030
-
2031
-
2032
-
2033
-
2034
- ### Why _ Not_ use HTML5 ` <template> ` ??
2027
+ ### Why _ Not_ use HTML5 ` <template> ` Element ??
2035
2028
2036
2029
Templates are an _ awesome_ feature in HTML5 which
2037
2030
allow the creation of reusable markup!
0 commit comments