|
| 1 | +from tkinter import * |
| 2 | +import datetime |
| 3 | +import time |
| 4 | +import winsound |
| 5 | +from threading import * |
| 6 | + |
| 7 | + |
| 8 | +root = Tk() |
| 9 | + |
| 10 | +root.geometry("400x200") |
| 11 | + |
| 12 | +def Threading(): |
| 13 | + t1=Thread(target=alarm) |
| 14 | + t1.start() |
| 15 | + |
| 16 | +def alarm(): |
| 17 | + |
| 18 | + while True: |
| 19 | + |
| 20 | + set_alarm_time = f"{hour.get()}:{minute.get()}:{second.get()}" |
| 21 | + |
| 22 | + |
| 23 | + time.sleep(1) |
| 24 | + |
| 25 | + |
| 26 | + current_time = datetime.datetime.now().strftime("%H:%M:%S") |
| 27 | + print(current_time,set_alarm_time) |
| 28 | + |
| 29 | + |
| 30 | + if current_time == set_alarm_time: |
| 31 | + print("Time to Wake up") |
| 32 | + |
| 33 | + winsound.PlaySound("sound.wav",winsound.SND_ASYNC) |
| 34 | + |
| 35 | +Label(root,text="Alarm Clock",font=("Helvetica 20 bold"),fg="red").pack(pady=10) |
| 36 | +Label(root,text="Set Time",font=("Helvetica 15 bold")).pack() |
| 37 | + |
| 38 | +frame = Frame(root) |
| 39 | +frame.pack() |
| 40 | + |
| 41 | +hour = StringVar(root) |
| 42 | +hours = ('00', '01', '02', '03', '04', '05', '06', '07', |
| 43 | + '08', '09', '10', '11', '12', '13', '14', '15', |
| 44 | + '16', '17', '18', '19', '20', '21', '22', '23', '24' |
| 45 | + ) |
| 46 | +hour.set(hours[0]) |
| 47 | + |
| 48 | +hrs = OptionMenu(frame, hour, *hours) |
| 49 | +hrs.pack(side=LEFT) |
| 50 | + |
| 51 | +minute = StringVar(root) |
| 52 | +minutes = ('00', '01', '02', '03', '04', '05', '06', '07', |
| 53 | + '08', '09', '10', '11', '12', '13', '14', '15', |
| 54 | + '16', '17', '18', '19', '20', '21', '22', '23', |
| 55 | + '24', '25', '26', '27', '28', '29', '30', '31', |
| 56 | + '32', '33', '34', '35', '36', '37', '38', '39', |
| 57 | + '40', '41', '42', '43', '44', '45', '46', '47', |
| 58 | + '48', '49', '50', '51', '52', '53', '54', '55', |
| 59 | + '56', '57', '58', '59', '60') |
| 60 | +minute.set(minutes[0]) |
| 61 | + |
| 62 | +mins = OptionMenu(frame, minute, *minutes) |
| 63 | +mins.pack(side=LEFT) |
| 64 | + |
| 65 | +second = StringVar(root) |
| 66 | +seconds = ('00', '01', '02', '03', '04', '05', '06', '07', |
| 67 | + '08', '09', '10', '11', '12', '13', '14', '15', |
| 68 | + '16', '17', '18', '19', '20', '21', '22', '23', |
| 69 | + '24', '25', '26', '27', '28', '29', '30', '31', |
| 70 | + '32', '33', '34', '35', '36', '37', '38', '39', |
| 71 | + '40', '41', '42', '43', '44', '45', '46', '47', |
| 72 | + '48', '49', '50', '51', '52', '53', '54', '55', |
| 73 | + '56', '57', '58', '59', '60') |
| 74 | +second.set(seconds[0]) |
| 75 | + |
| 76 | +secs = OptionMenu(frame, second, *seconds) |
| 77 | +secs.pack(side=LEFT) |
| 78 | + |
| 79 | +Button(root,text="Set Alarm",font=("Helvetica 15"),command=Threading).pack(pady=20) |
| 80 | + |
| 81 | + |
| 82 | +root.mainloop() |
| 83 | + |
| 84 | + |
| 85 | +Output:- |
| 86 | +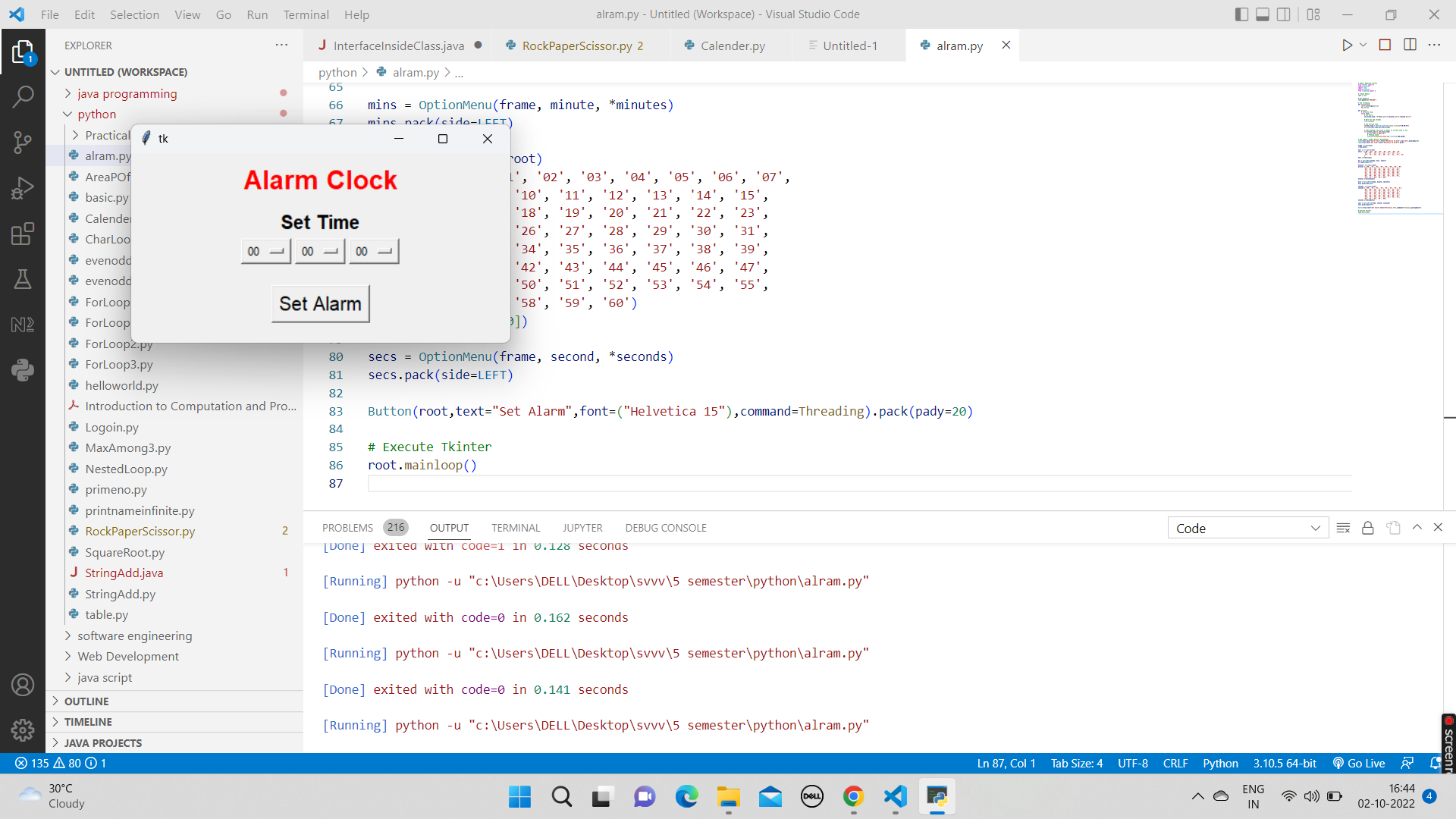 |
0 commit comments