|
1 |
| -# Fullstack Example with SvelteKit Actions and Load Functions |
| 1 | +# SvelteKit Example |
2 | 2 |
|
3 |
| -This example shows how to implement a **fullstack app in TypeScript with [SvelteKit](https://kit.svelte.dev/)** using SvelteKit's [actions](https://kit.svelte.dev/docs/form-actions) and [load](https://kit.svelte.dev/docs/form-actions#loading-data) functions and [Prisma Client](https://www.prisma.io/docs/reference/tools-and-interfaces/prisma-client). It uses a SQLite database file with some initial dummy data which you can find at [`./prisma/dev.db`](./prisma/dev.db). |
| 3 | +This example shows how to implement a simple web app using [SvelteKit](https://svelte.dev/docs/kit) and [Prisma ORM](https://www.prisma.io/docs). |
4 | 4 |
|
5 | 5 | ## Getting started
|
6 | 6 |
|
7 |
| -### 1. Download example and navigate into the project directory |
| 7 | +### 1. Download the example and navigate to the project directory |
8 | 8 |
|
9 | 9 | Download this example:
|
10 | 10 |
|
11 | 11 | ```
|
12 | 12 | npx try-prisma@latest --template orm/sveltekit
|
13 | 13 | ```
|
14 | 14 |
|
15 |
| -Then, navigate into the project directory: |
| 15 | +Then navigate to the project directory |
16 | 16 |
|
17 | 17 | ```
|
18 | 18 | cd sveltekit
|
@@ -41,281 +41,52 @@ This example uses a local SQLite database by default. If you want to use to [Pri
|
41 | 41 |
|
42 | 42 | 1. Set up a new Prisma Postgres instance in the Prisma Data Platform [Console](https://console.prisma.io) and copy the database connection URL.
|
43 | 43 | 2. Update the `datasource` block to use `postgresql` as the `provider` and paste the database connection URL as the value for `url`:
|
44 |
| - ```prisma |
45 |
| - datasource db { |
46 |
| - provider = "postgresql" |
47 |
| - url = "prisma+postgres://accelerate.prisma-data.net/?api_key=ey...." |
48 |
| - } |
49 |
| - ``` |
50 |
| -
|
51 |
| - > **Note**: In production environments, we recommend that you set your connection URL via an [environment variable](https://www.prisma.io/docs/orm/more/development-environment/environment-variables/managing-env-files-and-setting-variables), e.g. using a `.env` file. |
52 |
| -3. Install the Prisma Accelerate extension: |
53 |
| - ``` |
54 |
| - npm install @prisma/extension-accelerate |
55 |
| - ``` |
56 |
| -4. Add the Accelerate extension to the `PrismaClient` instance: |
57 |
| - ```diff |
58 |
| - + import { withAccelerate } from "@prisma/extension-accelerate" |
59 |
| -
|
60 |
| - + const prisma = new PrismaClient().$extends(withAccelerate()) |
61 |
| - ``` |
62 |
| -
|
63 |
| -That's it, your project is now configured to use Prisma Postgres! |
64 |
| -
|
65 |
| -### 2. Create and seed the database |
66 |
| -
|
67 |
| -Run the following command to create your database. This also creates the `User` and `Post` tables that are defined in [`prisma/schema.prisma`](./prisma/schema.prisma): |
68 |
| -
|
69 |
| -``` |
70 |
| -npx prisma migrate dev --name init |
71 |
| -``` |
72 |
| -
|
73 |
| -When `npx prisma migrate dev` is executed against a newly created database, seeding is also triggered. The seed file in [`prisma/seed.ts`](./prisma/seed.ts) will be executed and your database will be populated with the sample data. |
74 |
| -
|
75 |
| -**If you switched to Prisma Postgres in the previous step**, you need to trigger seeding manually (because Prisma Postgres already created an empty database instance for you, so seeding isn't triggered): |
76 |
| -
|
77 |
| -``` |
78 |
| -npx prisma db seed |
79 |
| -``` |
80 |
| -
|
81 |
| -
|
82 |
| -### 3. Start the app |
83 |
| -
|
84 |
| -``` |
85 |
| -npm run dev |
86 |
| -``` |
87 |
| -
|
88 |
| -The app is now running, navigate to [`http://localhost:5173/`](http://localhost:5173/) in your browser to explore its UI. |
89 |
| -
|
90 |
| -<details><summary>Expand for a tour through the UI of the app</summary> |
91 |
| -
|
92 |
| -<br /> |
93 |
| -
|
94 |
| -**Blog** (located in [`./src/routes/+page.svelte`](./src/routes/+page.svelte)) |
95 |
| -
|
96 |
| -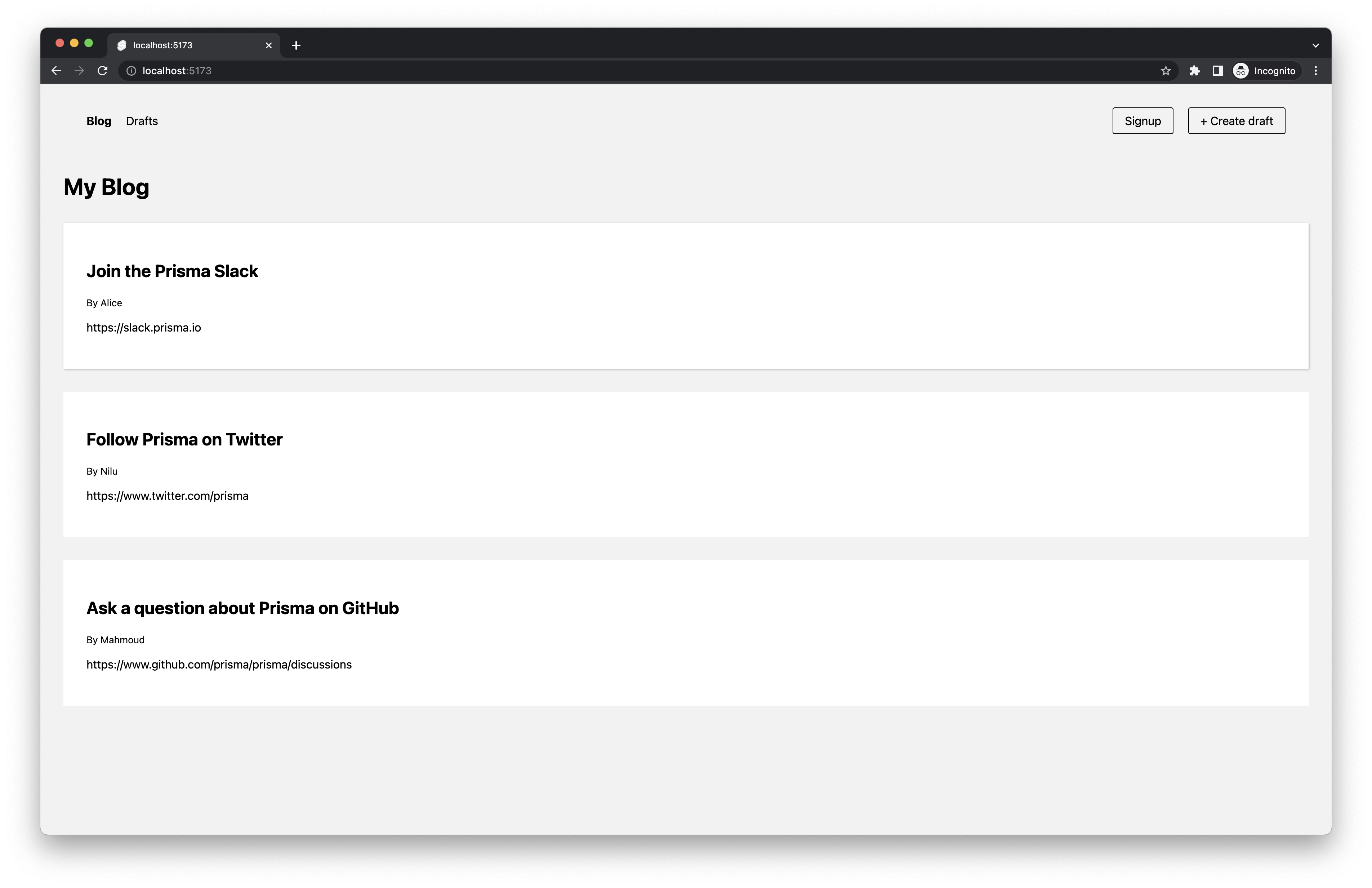 |
97 |
| -
|
98 |
| -**Signup** (located in [`./src/routes/signup/+page.svelte`](./src/routes/signup/+page.svelte)) |
99 |
| -
|
100 |
| -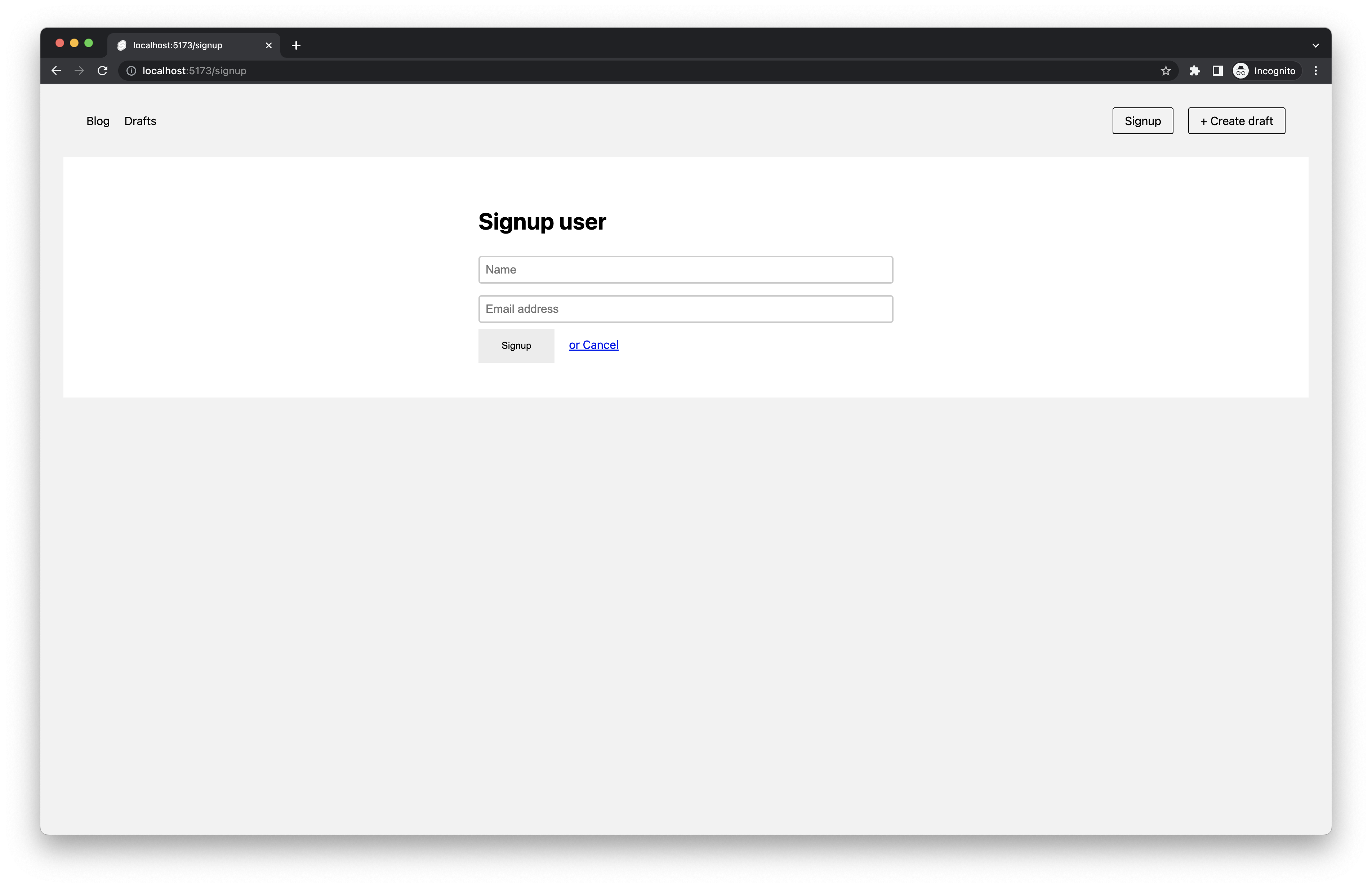 |
101 |
| -
|
102 |
| -**Create post (draft)** (located in [`./src/routes/create/+page.svelte`](./src/routes/create/+page.svelte)) |
103 |
| -
|
104 |
| -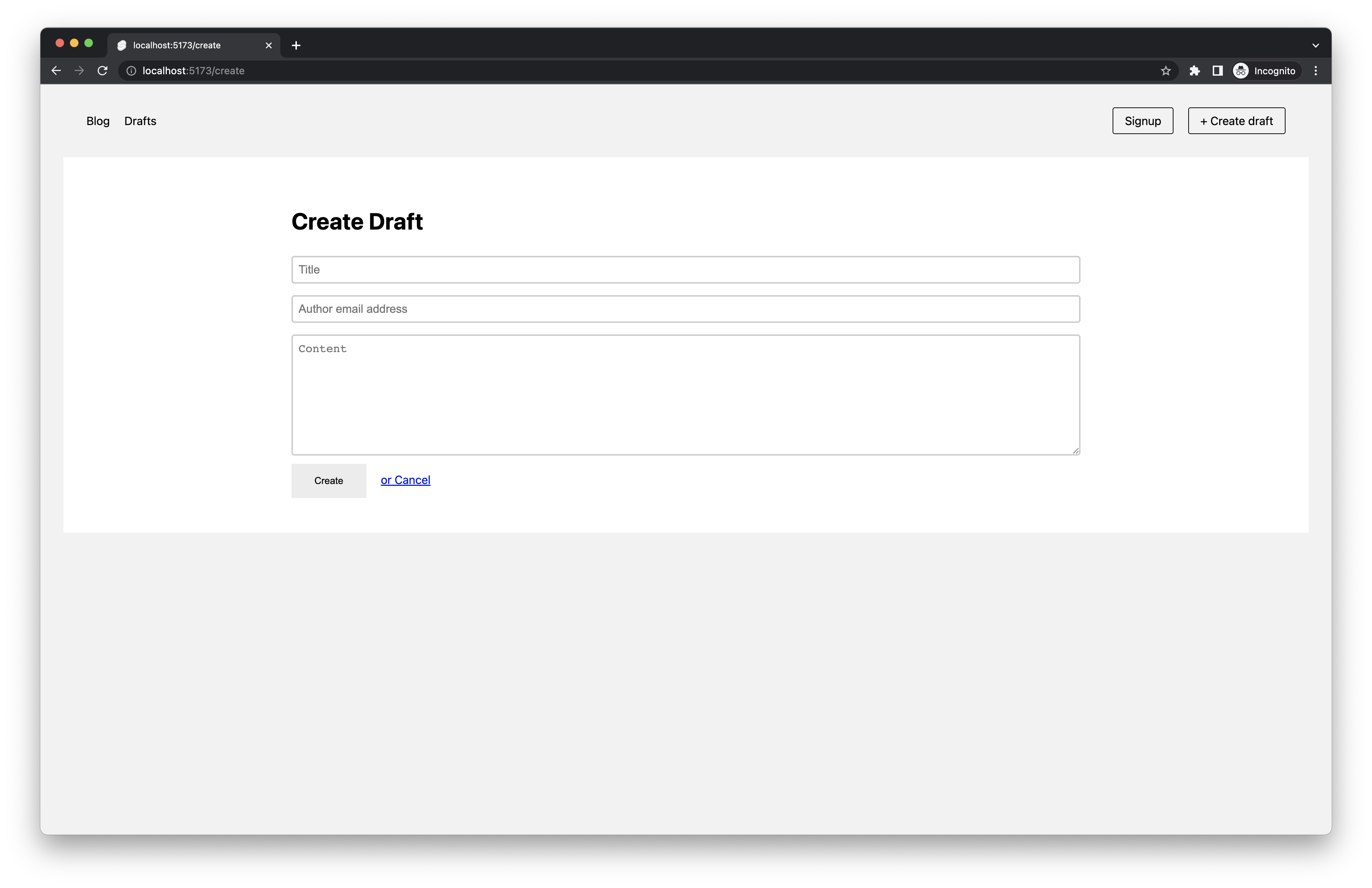 |
105 |
| -
|
106 |
| -**Drafts** (located in [`./src/routes/drafts/+page.svelte`](./src/routes/drafts/+page.svelte)) |
107 |
| -
|
108 |
| -![View Draft[(https://user-images.githubusercontent.com/49971500/214608068-8a8b2b12-f47b-434f-b668-14fdd1df9edd.png) |
109 |
| -
|
110 |
| -**View post** (located in [`./src/routes/p/[id]/+page.svelte`](./src/routes/p/[id]/+page.svelte)) (delete or publish here) |
111 |
| -
|
112 |
| -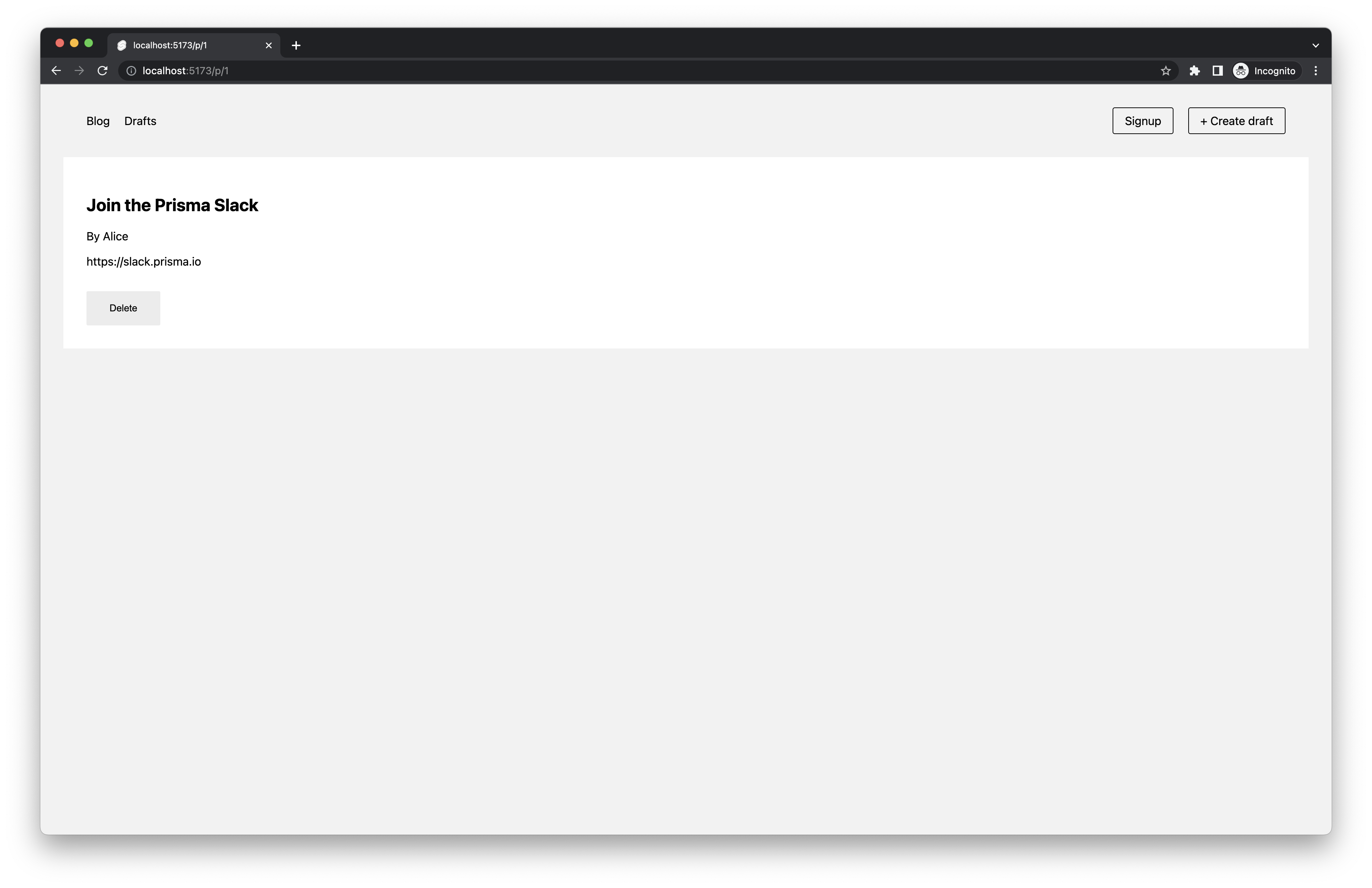 |
113 |
| -
|
114 |
| -</details> |
115 |
| -
|
116 |
| -## Using the SvelteKit Actions and Load functions |
117 |
| -
|
118 |
| -The `load` functions interact with the server to get data into your pages while the `actions` function mutates your data. Both these functions are defined in the `+page.server.ts` in the respective route folders. |
119 |
| -
|
120 |
| -### `LOAD` |
121 |
| -- `/`: Fetch all *published* posts |
122 |
| -- `/drafts`: Fetch all *drafted* posts |
123 |
| -- `/p/:id`: Fetch a *single* post by its `id` |
124 |
| -
|
125 |
| -### `ACTIONS` |
126 |
| -- `/create`: Create a new post |
127 |
| - - `default` action body: |
128 |
| - - `title: String` (required): The title of the post |
129 |
| - - `content: String` (required): The content of the post |
130 |
| - - `authorEmail: String` (required): The email post's author |
131 |
| -- `/p/:id`: |
132 |
| - - `publishPost` action: Publish a post by its `id` |
133 |
| - - `deletePost` action: Delete a post by its `id` |
134 |
| -- `/signup`: Create a new user |
135 |
| - - `default` action body: |
136 |
| - - `email: String` (required): The email address of the user |
137 |
| - - `name: String` (required): The name of the user |
138 |
| -
|
139 |
| -## Evolving the app |
140 |
| -
|
141 |
| -Evolving the application typically requires three steps: |
142 |
| -
|
143 |
| -1. Migrate your database using Prisma Migrate |
144 |
| -1. Update your server-side application code |
145 |
| -1. Build new UI features in Svelte |
146 |
| -
|
147 |
| -For the following example scenario, assume you want to add a "profile" feature to the app where users can create a profile and write a short bio about themselves. |
148 |
| -
|
149 |
| -### 1. Migrate your database using Prisma Migrate |
150 |
| -
|
151 |
| -The first step is to add a new table, e.g. called `Profile`, to the database. You can do this by adding a new model to your [Prisma schema file](./prisma/schema.prisma) file and then running a migration afterwards: |
152 |
| -
|
153 |
| -```diff |
154 |
| -// schema.prisma |
155 |
| -
|
156 |
| -model Post { |
157 |
| - id Int @default(autoincrement()) @id |
158 |
| - title String |
159 |
| - content String? |
160 |
| - published Boolean @default(false) |
161 |
| - author User? @relation(fields: [authorId], references: [id]) |
162 |
| - authorId Int |
163 |
| -} |
164 |
| -
|
165 |
| -model User { |
166 |
| - id Int @default(autoincrement()) @id |
167 |
| - name String? |
168 |
| - email String @unique |
169 |
| - posts Post[] |
170 |
| -+ profile Profile? |
171 |
| -} |
172 |
| -
|
173 |
| -+model Profile { |
174 |
| -+ id Int @default(autoincrement()) @id |
175 |
| -+ bio String? |
176 |
| -+ userId Int @unique |
177 |
| -+ user User @relation(fields: [userId], references: [id]) |
178 |
| -+} |
179 |
| -``` |
180 |
| - |
181 |
| -Once you've updated your data model, you can execute the changes against your database with the following command: |
182 |
| - |
183 |
| -``` |
184 |
| -npx prisma migrate dev |
185 |
| -``` |
186 |
| - |
187 |
| -### 2. Update your application code |
188 |
| - |
189 |
| -You can now use your `PrismaClient` instance to perform operations against the new `Profile` table. Here are some examples: |
190 |
| - |
191 |
| -#### Create a new profile for an existing user |
192 |
| - |
193 |
| -```ts |
194 |
| -const profile = await prisma.profile.create({ |
195 |
| - data: { |
196 |
| - bio: "Hello World", |
197 |
| - user: { |
198 |
| - connect: { email: "[email protected]" }, |
199 |
| - }, |
200 |
| - }, |
201 |
| -}); |
202 |
| -``` |
203 |
| - |
204 |
| -#### Create a new user with a new profile |
205 |
| - |
206 |
| -```ts |
207 |
| -const user = await prisma.user.create({ |
208 |
| - data: { |
209 |
| - |
210 |
| - name: "John", |
211 |
| - profile: { |
212 |
| - create: { |
213 |
| - bio: "Hello World", |
214 |
| - }, |
215 |
| - }, |
216 |
| - }, |
217 |
| -}); |
218 |
| -``` |
219 |
| - |
220 |
| -#### Update the profile of an existing user |
221 |
| - |
222 |
| -```ts |
223 |
| -const userWithUpdatedProfile = await prisma.user.update({ |
224 |
| - where: { email: "[email protected]" }, |
225 |
| - data: { |
226 |
| - profile: { |
227 |
| - update: { |
228 |
| - bio: "Hello Friends", |
229 |
| - }, |
230 |
| - }, |
231 |
| - }, |
232 |
| -}); |
233 |
| -``` |
234 |
| - |
235 |
| - |
236 |
| -### 3. Build new UI features in Svelte |
237 | 44 |
|
238 |
| -Once you have added a new route to your app (e.g. `/profile/+page.server.ts` with respective load and action operations), you can start building a new UI component in Svelte. It could e.g. be called `/profile/+page.svelte` and would be located in the `src/routes` directory. |
| 45 | + ```prisma |
| 46 | + datasource db { |
| 47 | + provider = "postgresql" |
| 48 | + url = "prisma+postgres://accelerate.prisma-data.net/?api_key=ey...." |
| 49 | + } |
| 50 | + ``` |
239 | 51 |
|
240 |
| -In the application code, you can manipulate data using `actions` and populate the UI with the data you receive from the `load` function. |
| 52 | + > **Note**: In production environments, we recommend that you set your connection URL via an [environment variable](https://www.prisma.io/docs/orm/more/development-environment/environment-variables/managing-env-files-and-setting-variables), e.g. using a `.env` file. |
241 | 53 |
|
| 54 | +3. Install the Prisma Accelerate extension: |
| 55 | + ``` |
| 56 | + npm install @prisma/extension-accelerate |
| 57 | + ``` |
| 58 | +4. Add the Accelerate extension to the `PrismaClient` instance: |
242 | 59 |
|
243 |
| -## Switch to another database (e.g. PostgreSQL, MySQL, SQL Server, MongoDB) |
244 |
| - |
245 |
| -If you want to try this example with another database than SQLite, you can adjust the the database connection in [`prisma/schema.prisma`](./prisma/schema.prisma) by reconfiguring the `datasource` block. |
| 60 | + ```diff |
| 61 | + + import { withAccelerate } from "@prisma/extension-accelerate" |
246 | 62 |
|
247 |
| -Learn more about the different connection configurations in the [docs](https://www.prisma.io/docs/reference/database-reference/connection-urls). |
| 63 | + + const prisma = new PrismaClient().$extends(withAccelerate()) |
| 64 | + ``` |
248 | 65 |
|
249 |
| -<details><summary>Expand for an overview of example configurations with different databases</summary> |
| 66 | +That's it, your project is now configured to use Prisma Postgres! |
250 | 67 |
|
251 |
| -### PostgreSQL |
| 68 | +### 2. Generate Prisma Client |
252 | 69 |
|
253 |
| -For PostgreSQL, the connection URL has the following structure: |
| 70 | +Run the following command to generate the Prisma Client. This is what you will be using to interact with your database. |
254 | 71 |
|
255 |
| -```prisma |
256 |
| -datasource db { |
257 |
| - provider = "postgresql" |
258 |
| - url = "postgresql://USER:PASSWORD@HOST:PORT/DATABASE?schema=SCHEMA" |
259 |
| -} |
260 | 72 | ```
|
261 |
| - |
262 |
| -Here is an example connection string with a local PostgreSQL database: |
263 |
| - |
264 |
| -```prisma |
265 |
| -datasource db { |
266 |
| - provider = "postgresql" |
267 |
| - url = "postgresql://janedoe:mypassword@localhost:5432/notesapi?schema=public" |
268 |
| -} |
| 73 | +npx prisma generate |
269 | 74 | ```
|
270 | 75 |
|
271 |
| -### MySQL |
272 |
| - |
273 |
| -For MySQL, the connection URL has the following structure: |
| 76 | +### 3. Start the SvelteKit server |
274 | 77 |
|
275 |
| -```prisma |
276 |
| -datasource db { |
277 |
| - provider = "mysql" |
278 |
| - url = "mysql://USER:PASSWORD@HOST:PORT/DATABASE" |
279 |
| -} |
280 | 78 | ```
|
281 |
| - |
282 |
| -Here is an example connection string with a local MySQL database: |
283 |
| - |
284 |
| -```prisma |
285 |
| -datasource db { |
286 |
| - provider = "mysql" |
287 |
| - url = "mysql://janedoe:mypassword@localhost:3306/notesapi" |
288 |
| -} |
289 |
| -``` |
290 |
| - |
291 |
| -### Microsoft SQL Server |
292 |
| - |
293 |
| -Here is an example connection string with a local Microsoft SQL Server database: |
294 |
| - |
295 |
| -```prisma |
296 |
| -datasource db { |
297 |
| - provider = "sqlserver" |
298 |
| - url = "sqlserver://localhost:1433;initial catalog=sample;user=sa;password=mypassword;" |
299 |
| -} |
| 79 | +npm run dev |
300 | 80 | ```
|
301 | 81 |
|
302 |
| -### MongoDB |
303 |
| - |
304 |
| -Here is an example connection string with a local MongoDB database: |
| 82 | +The server is now running at http://localhost:5173 |
305 | 83 |
|
306 |
| -```prisma |
307 |
| -datasource db { |
308 |
| - provider = "mongodb" |
309 |
| - url = "mongodb://USERNAME:PASSWORD@HOST/DATABASE?authSource=admin&retryWrites=true&w=majority" |
310 |
| -} |
311 |
| -``` |
| 84 | +## Switch to another database |
312 | 85 |
|
313 |
| -</details> |
| 86 | +If you want to try this example with another database than SQLite, refer to the [Databases](https://www.prisma.io/docs/orm/overview/databases) section in our documentation |
314 | 87 |
|
315 | 88 | ## Next steps
|
316 | 89 |
|
317 | 90 | - Check out the [Prisma docs](https://www.prisma.io/docs)
|
318 |
| -- [Join our community on Discord](https://pris.ly/discord?utm_source=github&utm_medium=prisma_examples&utm_content=next_steps_section) to share feedback and interact with other users. |
319 |
| -- [Subscribe to our YouTube channel](https://pris.ly/youtube?utm_source=github&utm_medium=prisma_examples&utm_content=next_steps_section) for live demos and video tutorials. |
320 |
| -- [Follow us on X](https://pris.ly/x?utm_source=github&utm_medium=prisma_examples&utm_content=next_steps_section) for the latest updates. |
321 |
| -- Report issues or ask [questions on GitHub](https://pris.ly/github?utm_source=github&utm_medium=prisma_examples&utm_content=next_steps_section). |
| 91 | +- Share your feedback on the [Prisma Discord](https://pris.ly/discord/) |
| 92 | +- Create issues and ask questions on [GitHub](https://github.com/prisma/prisma/) |
0 commit comments