You signed in with another tab or window. Reload to refresh your session.You signed out in another tab or window. Reload to refresh your session.You switched accounts on another tab or window. Reload to refresh your session.Dismiss alert
8774: feat: Honor `.cargo/config.toml` r=matklad a=Veykril
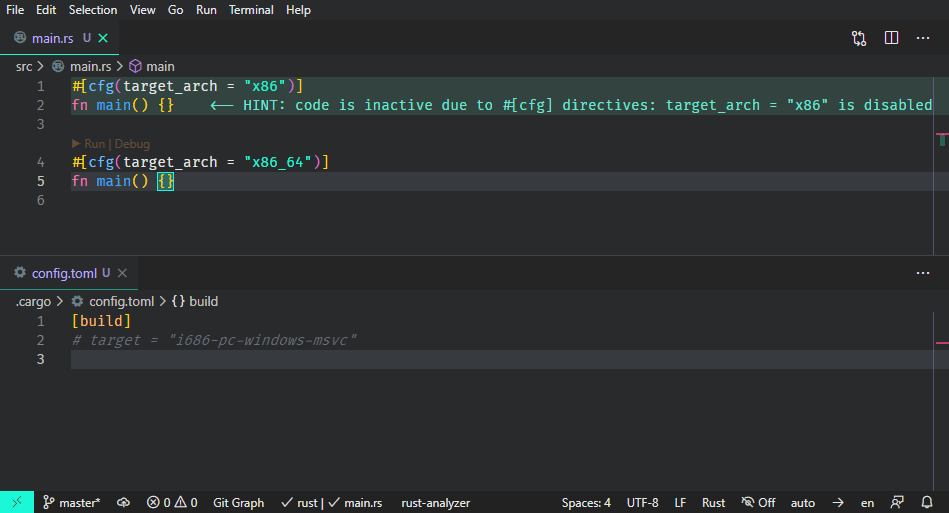
Implements `cargo/.config` build target and cfg access by using unstable cargo options:
- `cargo config get` to read the target triple out of the config to pass to `cargo metadata` --filter-platform
- `cargo rustc --print` to read out the `rustc_cfgs`, this causes us to honor `rustflags` and the like.
If those commands fail, due to not having a nightly toolchain present for example, they will fall back to invoking rustc directly as we currently do.
I personally think it should be fine to use these unstable options as they are unlikely to change(even if they did it shouldn't be a problem due to the fallback) and don't burden the user if they do not have a nightly toolchain at hand since we fall back to the previous behaviour.
cc #8741Closes#6604, Closes#5904, Closes#8430, Closes#8480
Co-authored-by: Lukas Wirth <[email protected]>
0 commit comments